Python program to reverse the content of a file and store it in another file
Last Updated :
20 Feb, 2023
Given a text file. The task is to reverse as well as stores the content from an input file to an output file.
This reversing can be performed in two types.
- Full reversing: In this type of reversing all the content gets reversed.
- Word to word reversing: In this kind of reversing the last word comes first and the first word goes to the last position.
Example 1: Full Reversing
Input: Hello Geeks
for geeks!
Output:!skeeg rof
skeeG olleH
Example 2: Word to word reversing
Input:
Hello Geeks
for geeks!
Output:
geeks! for
Geeks Hello
Example 1: Full Reversing
Text file:
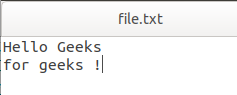
Python
f1 = open ( "output1.txt" , "w" )
with open ( "file.txt" , "r" ) as myfile:
data = myfile.read()
data_1 = data[:: - 1 ]
f1.write(data_1)
f1.close()
|
Output:
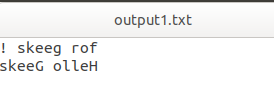
Time complexity: O(n), where n is the length of the input file “file.txt”.
Auxiliary space: O(n), where n is the length of the input file “file.txt”.
Example 2: Reversing the order of lines. We will use the above text file as input.
Python3
f2 = open ( "output2.txt" , "w" )
with open ( "file.txt" , "r" ) as myfile:
data = myfile.readlines()
data_2 = data[:: - 1 ]
f2.writelines(data_2)
f2.close()
|
Output:
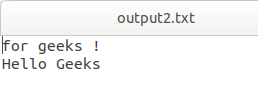
Please Login to comment...