String Slicing in Python
Last Updated :
27 Mar, 2023
Python slicing is about obtaining a sub-string from the given string by slicing it respectively from start to end.
How String slicing in Python works
For understanding slicing we will use different methods, here we will cover 2 methods of string slicing, one using the in-build slice() method and another using the [:] array slice. String slicing in Python is about obtaining a sub-string from the given string by slicing it respectively from start to end.
Python slicing can be done in two ways:
- Using a slice() method
- Using the array slicing [:: ] method
Index tracker for positive and negative index: String indexing and slicing in python. Here, the Negative comes into consideration when tracking the string in reverse.
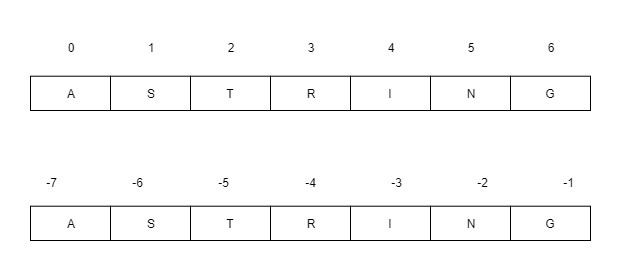
Method 1: Using the slice() method
The slice() constructor creates a slice object representing the set of indices specified by range(start, stop, step).
Syntax:
- slice(stop)
- slice(start, stop, step)
Parameters: start: Starting index where the slicing of object starts. stop: Ending index where the slicing of object stops. step: It is an optional argument that determines the increment between each index for slicing. Return Type: Returns a sliced object containing elements in the given range only.
Example:
Python3
String = 'ASTRING'
s1 = slice ( 3 )
s2 = slice ( 1 , 5 , 2 )
s3 = slice ( - 1 , - 12 , - 2 )
print ( "String slicing" )
print (String[s1])
print (String[s2])
print (String[s3])
|
Output:
String slicing
AST
SR
GITA
Method 2: Using the List/array slicing [ :: ] method
In Python, indexing syntax can be used as a substitute for the slice object. This is an easy and convenient way to slice a string using list slicing and Array slicing both syntax-wise and execution-wise. A start, end, and step have the same mechanism as the slice() constructor.
Below we will see string slicing in Python with examples.
Syntax
arr[start:stop] # items start through stop-1
arr[start:] # items start through the rest of the array
arr[:stop] # items from the beginning through stop-1
arr[:] # a copy of the whole array
arr[start:stop:step] # start through not past stop, by step
Example 1:
In this example, we will see slicing in python list the index start from 0 indexes and ending with a 2 index(stops at 3-1=2 ).
Python3
String = 'GEEKSFORGEEKS'
print (String[: 3 ])
|
Output:
GEE
Example 2:
In this example, we will see the example of starting from 1 index and ending with a 5 index(stops at 3-1=2 ), and the skipping step is 2. It is a good example of Python slicing string by character.
Python3
String = 'GEEKSFORGEEKS'
print (String[ 1 : 5 : 2 ])
|
Output:
EK
Example 3:
In this example, we will see the example of starting from -1 indexes and ending with a -12 index(stops at 3-1=2 )and the skipping step is -2.
Python3
String = 'GEEKSFORGEEKS'
print (String[ - 1 : - 12 : - 2 ])
|
Output:
SEGOSE
Example 4:
In this example, the whole string is printed in reverse order.
Python3
String = 'GEEKSFORGEEKS'
print (String[:: - 1 ])
|
Output:
SKEEGROFSKEEG
Note: To know more about strings click here.
Using islice()
The islice() is a built-in function defined in itertools module. It is used to get an iterator which is an index-based slicing of any iterable. It works like a standard slice but returns an iterator.
Syntax:
itertools.islice(iterable, start, stop[, step])
Parameters: iterable: Any iterable sequence like list, string, tuple etc. start: The start index from where the slicing of iterable starts. stop: The end index from where the slicing of iterable ends. step: An optional argument. It specifies the gap between each index for slicing. Return Type: Return an iterator from the given iterable sequence.
Example:
Python3
import itertools
String = 'GEEKSFORGEEKS'
print (''.join(itertools.islice(String, 3 , 7 )))
|
Please Login to comment...