Python If Else on One Line
Last Updated :
08 May, 2024
The if-elif-else statement is used in Python for decision-making i.e. the program will evaluate the test expression and execute the remaining statements only if the given test expression turns out to be true. This allows validation for multiple expressions. This article will show how the traditional if…elif…else statement differs from If Elif in Python.
Python Shorthandf If Else
In the traditional if elif else statement in Python, the conditions are written in different blocks of code, and each block of code is indented according to the parent if condition. It is mostly used when there is a multiple-line code, which makes it easier to understand.
Syntax of if elif else statement
if (condition):
statement
elif (condition):
statement
else:
statement
Example: In this example, we will find if a number if positive, negative or zero using the if-elif-else statement.
Python
x = 0
# traditional python if elif else statement
if x > 0:
print("Positive")
elif x < 0:
print("Negative")
else:
print("Zero")
Output:
Zero
The concept can also be implemented using the short-hand method using Python Ternary Operation.
One Liner if elif else Statements
The one-liner if elif else statement in Python are used when there are a simple and straightforward conditions to be implemented. This means that the code can be fitted in a single line expression. It uses a Python dictionary like structure along with Python dictionary get() method.
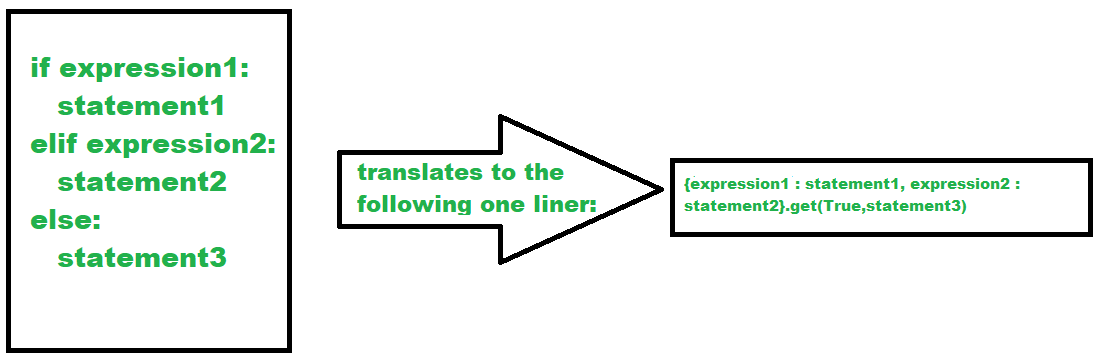
Python if elif else statement structure
Syntax of Python one-liner if elif else Statement:
This can be easily interpreted as if condition 1 is True run code 1 if condition 2 is True run code 2 and if both of them are false run the third code.
{(condition1 : Â <code1>) , (condition2 : Â <code2>) }.get(True, <code3>)
Example: In this example, we will find if a number if positive, negative or zero. First, we declared a number in ‘x’ variable. Then we used one liner python if elif else statement to check the three conditions.
Python
x = 0
# Python one liner if elif else statement
result = {x > 0: "Positive", x < 0: "Negative"}.get(True, "Zero")
print(result)
Output:
Zero
Note: There are a few important things to keep in mind while using one liner for python if elif else statement. One of them is that, it works on the concept of python dictionary. This means the conditions are stored in the form of dictionary keys and the statement to be executed is stored in the form of dictionary values. One the keys, that is the condition returns True, only then the value of the corresponding key is executed.
Example: In this code we provide conditions as the dictionary keys, and the code to execute as the values. But this code won’t get you the desired results for this syntax of if-elif-else in Python. It will evaluate all three conditions and perform its corresponding action.
Python
x = 0
{x > 0: print("Positive"), x < 0: print("Negative")}.get(True, print("Zero"))
Output:
Positive
Negative
Zero
Please Login to comment...