*args and **kwargs in Python
Last Updated :
19 Jun, 2024
In this article, we will cover what ** (double star/asterisk) and * (star/asterisk) do for parameters in Python, Here, we will also cover args and kwargs examples in Python. We can pass a variable number of arguments to a function using special symbols.
There are two special symbols:
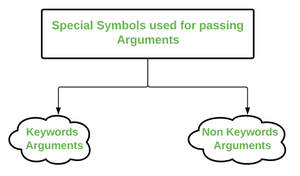
*args and **kwargs in Python
Special Symbols Used for passing arguments in Python:
- *args (Non-Keyword Arguments)
- **kwargs (Keyword Arguments)
Note: “We use the “wildcard” or “*” notation like this – *args OR **kwargs – as our function’s argument when we have doubts about the number of arguments we should pass in a function.”
What is Python *args?
The special syntax *args in function definitions in Python is used to pass a variable number of arguments to a function. It is used to pass a non-keyworded, variable-length argument list.
- The syntax is to use the symbol * to take in a variable number of arguments; by convention, it is often used with the word args.
- What *args allows you to do is take in more arguments than the number of formal arguments that you previously defined. With *args, any number of extra arguments can be tacked on to your current formal parameters (including zero extra arguments).
- For example, we want to make a multiply function that takes any number of arguments and is able to multiply them all together. It can be done using *args.
- Using the *, the variable that we associate with the * becomes iterable meaning you can do things like iterate over it, run some higher-order functions such as map and filter, etc.
Example 1:
Python program to illustrate *args for a variable number of arguments
python
def myFun(*argv):
for arg in argv:
print(arg)
myFun('Hello', 'Welcome', 'to', 'GeeksforGeeks')
Output:
Hello
Welcome
to
GeeksforGeeks
Example 2:
Python program to illustrate *args with a first extra argument
Python
def myFun(arg1, *argv):
print("First argument :", arg1)
for arg in argv:
print("Next argument through *argv :", arg)
myFun('Hello', 'Welcome', 'to', 'GeeksforGeeks')
Output:
First argument : Hello
Next argument through *argv : Welcome
Next argument through *argv : to
Next argument through *argv : GeeksforGeeks
What is Python **kwargs?
The special syntax **kwargs in function definitions in Python is used to pass a keyworded, variable-length argument list. We use the name kwargs with the double star. The reason is that the double star allows us to pass through keyword arguments (and any number of them).
- A keyword argument is where you provide a name to the variable as you pass it into the function.
- One can think of the kwargs as being a dictionary that maps each keyword to the value that we pass alongside it. That is why when we iterate over the kwargs there doesn’t seem to be any order in which they were printed out.
Example 1:
Python program to illustrate *kwargs for a variable number of keyword arguments. Here **kwargs accept keyworded variable-length argument passed by the function call. for first=’Geeks’ first is key and ‘Geeks’ is a value. in simple words, what we assign is value, and to whom we assign is key.
Python
def myFun(**kwargs):
for key, value in kwargs.items():
print("%s == %s" % (key, value))
# Driver code
myFun(first='Geeks', mid='for', last='Geeks')
Output:
first == Geeks
mid == for
last == Geeks
Example 2:
Python program to illustrate **kwargs for a variable number of keyword arguments with one extra argument. All the same, but one change is we passing non-keyword argument which acceptable by positional argument(arg1 in myFun). and keyword arguments we passing are acceptable by **kwargs. simple right?
Python
def myFun(arg1, **kwargs):
for key, value in kwargs.items():
print("%s == %s" % (key, value))
# Driver code
myFun("Hi", first='Geeks', mid='for', last='Geeks')
Output:
first == Geeks
mid == for
last == Geeks
Using both *args and **kwargs in Python to call a function
Example 1:
Here, we are passing *args and **kwargs as an argument in the myFun function. Passing *args to myFun simply means that we pass the positional and variable-length arguments which are contained by args. so, “Geeks” pass to the arg1 , “for” pass to the arg2, and “Geeks” pass to the arg3. When we pass **kwargs as an argument to the myFun it means that it accepts keyword arguments. Here, “arg1” is key and the value is “Geeks” which is passed to arg1, and just like that “for” and “Geeks” pass to arg2 and arg3 respectively. After passing all the data we are printing all the data in lines.
python
def myFun(arg1, arg2, arg3):
print("arg1:", arg1)
print("arg2:", arg2)
print("arg3:", arg3)
# Now we can use *args or **kwargs to
# pass arguments to this function :
args = ("Geeks", "for", "Geeks")
myFun(*args)
kwargs = {"arg1": "Geeks", "arg2": "for", "arg3": "Geeks"}
myFun(**kwargs)
Output:
arg1: Geeks
arg2: for
arg3: Geeks
arg1: Geeks
arg2: for
arg3: Geeks
Example 2:
Here, we are passing *args and **kwargs as an argument in the myFun function. where ‘geeks’, ‘for’, ‘geeks’ is passed as *args, and first=”Geeks”, mid=”for”, last=”Geeks” is passed as **kwargs and printing in the same line.
python
def myFun(*args, **kwargs):
print("args: ", args)
print("kwargs: ", kwargs)
# Now we can use both *args ,**kwargs
# to pass arguments to this function :
myFun('geeks', 'for', 'geeks', first="Geeks", mid="for", last="Geeks")
Output:
args: ('geeks', 'for', 'geeks')
kwargs: {'first': 'Geeks', 'mid': 'for', 'last': 'Geeks'}
Using *args and **kwargs in Python to set values of object
- *args receives arguments as a tuple.
- **kwargs receives arguments as a dictionary.
Example 1: using Python *args
Python
# defining car class
class Car():
# args receives unlimited no. of arguments as an array
def __init__(self, *args):
# access args index like array does
self.speed = args[0]
self.color = args[1]
# creating objects of car class
audi = Car(200, 'red')
bmw = Car(250, 'black')
mb = Car(190, 'white')
# printing the color and speed of the cars
print(audi.color)
print(bmw.speed)
Output:
red
250
Example 2: using Python **kwargs
Python
# defining car class
class Car():
# args receives unlimited no. of arguments as an array
def __init__(self, **kwargs):
# access args index like array does
self.speed = kwargs['s']
self.color = kwargs['c']
# creating objects of car class
audi = Car(s=200, c='red')
bmw = Car(s=250, c='black')
mb = Car(s=190, c='white')
# printing the color and speed of cars
print(audi.color)
print(bmw.speed)
Output:
red
250
*args and **kwargs in Python – FAQs
Why use *args
and **kwargs
in Python?
*args
and *
*kwargs
allow functions to accept a variable number of arguments:
*args
(arguments) allows you to pass a variable number of positional arguments to a function.**kwargs
(keyword arguments) allows you to pass a variable number of keyword arguments (key-value pairs) to a function.
Difference between *args
and **kwargs
in Python?
*args collects additional positional arguments as a tuple, while **kwargs collects additional keyword arguments as a dictionary.
def example_function(*args, **kwargs):
print(args) # tuple of positional arguments
print(kwargs) # dictionary of keyword arguments
example_function(1, 2, 3, name='Alice', age=30)
Output:
(1, 2, 3)
{'name': 'Alice', 'age': 30}
Is *args
a list or tuple in Python?
*args collects additional positional arguments into a tuple, not a list. The arguments are accessible using tuple indexing and iteration.
def example_function(*args):
print(type(args)) # <class 'tuple'>
example_function(1, 2, 3)
Why use *args
instead of a list?
*args is used when you want to pass a variable number of arguments to a function without explicitly specifying each argument in a list. It allows for flexibility and simplicity when defining functions that may take an unknown number of arguments.
def sum_values(*args):
total = sum(args)
return total
result = sum_values(1, 2, 3, 4, 5)
print(result) # Output: 15
How to pass **kwargs
?
To pass keyword arguments (**kwargs) to a function, you provide key-value pairs when calling the function.
def example_function(**kwargs):
print(kwargs)
example_function(name='Alice', age=30)
Output:
{'name': 'Alice', 'age': 30}
Inside the function, kwargs
will be a dictionary containing the passed keyword arguments.
Please Login to comment...