Find a Mother Vertex in a Graph
Last Updated :
26 Feb, 2024
Write a function to find a mother vertex in the graph.
What is a Mother Vertex?Â
A mother vertex in a graph G = (V, E) is a vertex v such that all other vertices in G can be reached by a path from v
Example:Â
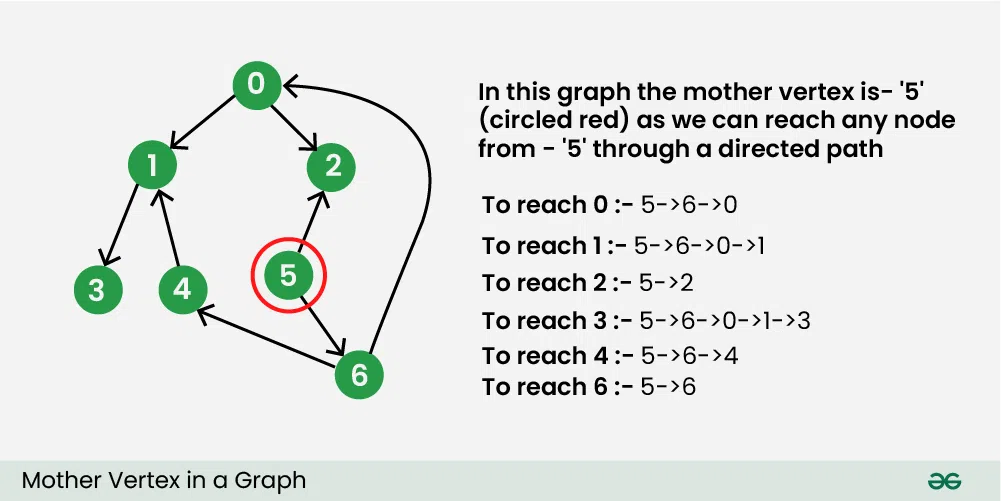
Input: Graph as shown above
Output: 5
Note: There can be more than one mother vertices in a graph. We need to output anyone of them.Â
For example, in the below graph, vertices 0, 1, and 2 are mother vertices.
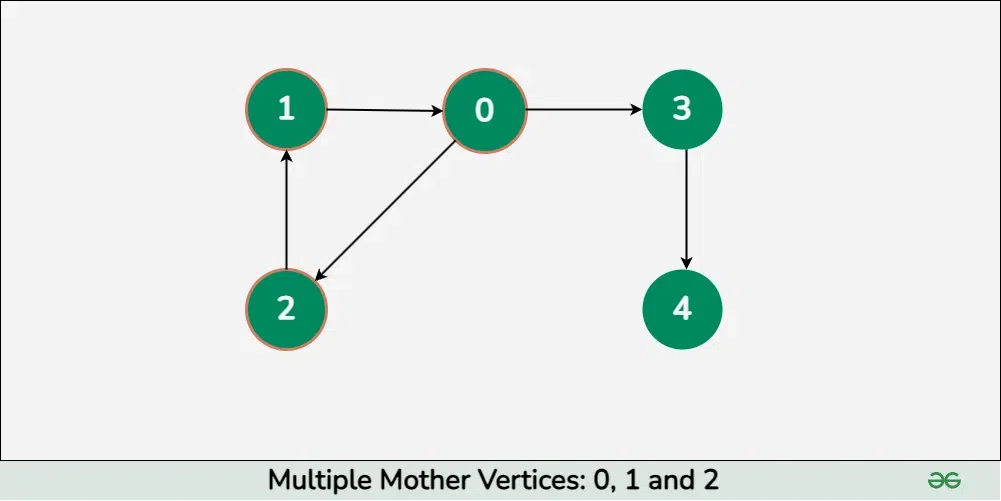
Naive Approach: To solve the problem follow the below idea:
A trivial approach will be to perform a DFS/BFS on all the vertices and find whether we can reach all the vertices from that vertex.Â
Time Complexity: O(V * (E+V))
Auxiliary Space: O(1) It will be O(V) if the stack space for DFS is considered or if we use a BFS.
To solve the problem follow the below idea:
The idea is based on Kosaraju’s Strongly Connected Component Algorithm. In a graph of strongly connected components, mother vertices are always vertices of the source components in the component graph. The idea is based on the below fact:
If there exists a mother vertex (or vertices), then one of the mother vertices is the last finished vertex in DFS. (Or a mother vertex has the maximum finish time in DFS traversal). A vertex is said to be finished in DFS if a recursive call for its DFS is over, i.e., all descendants of the vertex have been visited.Â
How does the above idea work?Â
Let the last finished vertex be v. Basically, we need to prove that there cannot be an edge from another vertex u to v if u is not another mother vertex (Or there cannot exist a non-mother vertex u such that u-?v is an edge). There can be two possibilities.
- A recursive DFS call is made for u before v. If an edge u-?v exists, then v must have finished before u because v is reachable through u and a vertex finishes after all its descendants.
- A recursive DFS call is made for v before u. In this case also, if an edge u-?v exists, then either v must finish before u (which contradicts our assumption that v is finished at the end) OR u should be reachable from v (which means u is another mother vertex).
Follow the given steps to solve the problem:
- Do DFS traversal of the given graph. While doing traversal keep track of the last finished vertex ‘v’. This step takes O(V+E) time.
- If there exists a mother vertex (or vertices), then v must be one (or one of them). Check if v is a mother vertex by doing DFS/BFS from v. This step also takes O(V+E) time.
Below is the implementation of the above approach.
C++
#include <bits/stdc++.h>
using namespace std;
class Graph {
int V;
list< int >* adj;
void DFSUtil( int v, vector< bool >& visited);
public :
Graph( int V);
void addEdge( int v, int w);
int findMother();
};
Graph::Graph( int V)
{
this ->V = V;
adj = new list< int >[V];
}
void Graph::DFSUtil( int v, vector< bool >& visited)
{
visited[v] = true ;
list< int >::iterator i;
for (i = adj[v].begin(); i != adj[v].end(); ++i)
if (!visited[*i])
DFSUtil(*i, visited);
}
void Graph::addEdge( int v, int w)
{
adj[v].push_back(w);
}
int Graph::findMother()
{
vector< bool > visited(V, false );
int v = 0;
for ( int i = 0; i < V; i++) {
if (visited[i] == false ) {
DFSUtil(i, visited);
v = i;
}
}
fill(visited.begin(), visited.end(), false );
DFSUtil(v, visited);
for ( int i = 0; i < V; i++)
if (visited[i] == false )
return -1;
return v;
}
int main()
{
Graph g(7);
g.addEdge(0, 1);
g.addEdge(0, 2);
g.addEdge(1, 3);
g.addEdge(4, 1);
g.addEdge(6, 4);
g.addEdge(5, 6);
g.addEdge(5, 2);
g.addEdge(6, 0);
cout << "A mother vertex is " << g.findMother();
return 0;
}
|
Java
import java.util.*;
class GFG {
static void addEdge( int u, int v,
ArrayList<ArrayList<Integer> > adj)
{
adj.get(u).add(v);
}
static void DFSUtil(ArrayList<ArrayList<Integer> > g,
int v, boolean [] visited)
{
visited[v] = true ;
for ( int x : g.get(v)) {
if (!visited[x]) {
DFSUtil(g, x, visited);
}
}
}
static int
motherVertex(ArrayList<ArrayList<Integer> > g, int V)
{
boolean [] visited = new boolean [V];
int v = - 1 ;
for ( int i = 0 ; i < V; i++) {
if (!visited[i]) {
DFSUtil(g, i, visited);
v = i;
}
}
boolean [] check = new boolean [V];
DFSUtil(g, v, check);
for ( boolean val : check) {
if (!val) {
return - 1 ;
}
}
return v;
}
public static void main(String[] args)
{
int V = 7 ;
int E = 8 ;
ArrayList<ArrayList<Integer> > adj
= new ArrayList<ArrayList<Integer> >();
for ( int i = 0 ; i < V; i++) {
adj.add( new ArrayList<Integer>());
}
addEdge( 0 , 1 , adj);
addEdge( 0 , 2 , adj);
addEdge( 1 , 3 , adj);
addEdge( 4 , 1 , adj);
addEdge( 6 , 4 , adj);
addEdge( 5 , 6 , adj);
addEdge( 5 , 2 , adj);
addEdge( 6 , 0 , adj);
System.out.println( "A mother vertex is "
+ motherVertex(adj, V));
}
}
|
Python3
from collections import defaultdict
class Graph:
def __init__( self , vertices):
self .V = vertices
self .graph = defaultdict( list )
def DFSUtil( self , v, visited):
visited[v] = True
for i in self .graph[v]:
if visited[i] = = False :
self .DFSUtil(i, visited)
def addEdge( self , v, w):
self .graph[v].append(w)
def findMother( self ):
visited = [ False ] * ( self .V)
v = 0
for i in range ( self .V):
if visited[i] = = False :
self .DFSUtil(i, visited)
v = i
visited = [ False ] * ( self .V)
self .DFSUtil(v, visited)
if any (i = = False for i in visited):
return - 1
else :
return v
if __name__ = = '__main__' :
g = Graph( 7 )
g.addEdge( 0 , 1 )
g.addEdge( 0 , 2 )
g.addEdge( 1 , 3 )
g.addEdge( 4 , 1 )
g.addEdge( 6 , 4 )
g.addEdge( 5 , 6 )
g.addEdge( 5 , 2 )
g.addEdge( 6 , 0 )
print ( "A mother vertex is " + str (g.findMother()))
|
C#
using System;
using System.Collections.Generic;
class GFG {
static void addEdge( int u, int v, List<List< int > > adj)
{
adj[u].Add(v);
}
static void DFSUtil(List<List< int > > g, int v,
bool [] visited)
{
visited[v] = true ;
foreach ( int x in g[v])
{
if (!visited[x]) {
DFSUtil(g, x, visited);
}
}
}
static int motherVertex(List<List< int > > g, int V)
{
bool [] visited = new bool [V];
int v = -1;
for ( int i = 0; i < V; i++) {
if (!visited[i]) {
DFSUtil(g, i, visited);
v = i;
}
}
bool [] check = new bool [V];
DFSUtil(g, v, check);
foreach ( bool val in check)
{
if (!val) {
return -1;
}
}
return v;
}
public static void Main(String[] args)
{
int V = 7;
List<List< int > > adj = new List<List< int > >();
for ( int i = 0; i < V; i++) {
adj.Add( new List< int >());
}
addEdge(0, 1, adj);
addEdge(0, 2, adj);
addEdge(1, 3, adj);
addEdge(4, 1, adj);
addEdge(6, 4, adj);
addEdge(5, 6, adj);
addEdge(5, 2, adj);
addEdge(6, 0, adj);
Console.WriteLine( "A mother vertex is "
+ motherVertex(adj, V));
}
}
|
Javascript
<script>
function addEdge(u, v, adj)
{
adj[u].push(v);
}
function DFSUtil(g, v, visited)
{
visited[v] = true ;
for (let x in g[v])
{
if (!visited[x])
{
DFSUtil(g, x, visited);
}
}
}
function motherVertex(g, V)
{
let visited = new Array(V);
for (let i = 0; i < V; i++)
{
visited[i] = false ;
}
let v = -1;
for (let i = 0; i < V; i++)
{
if (!visited[i])
{
DFSUtil(g, i, visited);
v = i;
}
}
let check = new Array(V);
for (let i = 0; i < V; i++)
{
check[i] = false ;
}
DFSUtil(g, v, check);
for (let val in check)
{
if (!val)
{
return -1;
}
}
return v-1;
}
let V = 7;
let E = 8;
let adj = [];
for (let i = 0; i < V; i++)
{
adj.push([]);
}
addEdge(0, 1,adj);
addEdge(0, 2,adj);
addEdge(1, 3,adj);
addEdge(4, 1,adj);
addEdge(6, 4,adj);
addEdge(5, 6,adj);
addEdge(5, 2,adj);
addEdge(6, 0,adj);
document.write( "A mother vertex is " + motherVertex(adj, V));
</script>
|
Output
A mother vertex is 5
Time Complexity: O(V + E)
Auxiliary Space: O(V)
Please Login to comment...