Implementing Configuration Versioning with Spring Cloud Config
Last Updated :
21 Jun, 2024
Configuration versioning is the critical aspect of maintaining and managing the configurations in the microservices architecture. It can allow the teams to track the changes, revert to previous versions and manage the configurations more efficiently, Spring Cloud Config can provide a powerful way to externalize configurations and supports the versioning through integration with version control systems like Git. This article will guide you through the process of implementing the configuration version using Spring Cloud Config of the Spring applications.
Prerequisites
- Basic understanding of the Spring Boot and Spring Cloud.
- The version control system is set like Git.
- Java Development Kit installed in your local system.
- Maven for the dependency management system.
Main Concept
Spring Cloud Config can provides the server and client-side supports for the externalized configuration in the distrubted system. With the Spring Cloud Config, we can manage the configurations in the central place and version it using the Git repository. This setup can allows the application to fetch their configuration from the server which can retrives it from the version-controlled repository.
Key Terminologies
- Configuration Repository: The central location where the configuration files are stored and managed of the application. In Spring Cloud Config, it can often the Git repository, the repository contains the configruation properties for the different applications and enviornments and it can enabling the version control and collaboration.
- Spring Cloud Config Server: The Spring Boot application that can provides the configuration properties to the client applications. It can acts as the middle between the configurations from the repository and serves them to the clients.
- Spring Cloud Config Client: The client applicatio that can be retrieves the configuration properties from Config Server. The Client application can be any Spring Boot application that needs the externalized configuration. It can fetches the configurations during the startup and can refresh them at runtime of the application.
- Git: The distributed system used to track the changes in the source code. In context of the Spring Cloud Config, Git can be used as the configuration repository to manage the configuration files, track changes and collaborate on the configuration management.
- Versioning: The process of the tracking and managing the changes to the configuration files. Versioning can allows the developers to maintain the history of configuration changes, roll back to the previous versions and understand the evolution of the configurations over time.
Implementing Configuration Versioning with Spring Cloud Config
Create the Git repository
We can create the git repository and add the application.properties file with the following content.
# application.properties
message=Hello from Config Server
Create the Config-Server
Step 1: Create the spring project using spring initializer and add the below required dependencies.
Dependencies:
- Spring Web
- Spring Dev Tools
- Lombok
- Spring Cloud Config
After creating the Spring project, the file structure will be like below image.
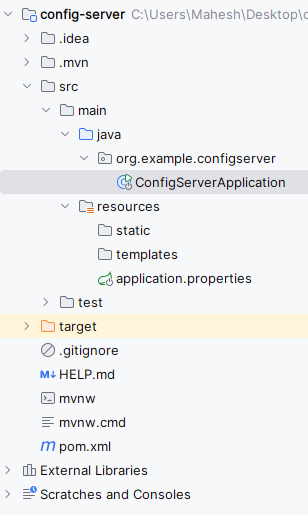
Step 2: Configure the Application Properties
Open the application.properties file and add the configuring the server port and git repository uri configuration of the application.
spring.application.name=config-server
spring.cloud.config.server.git.uri=https://github.com/yourusername/config-repo
server.port=8888
Step 3: Main Class
Open the main class, add the @EnableConfigServer to activate the Spring cloud config functionality of the application.
Java
package org.example.configserver;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.config.server.EnableConfigServer;
@SpringBootApplication
@EnableConfigServer
public class ConfigServerApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigServerApplication.class, args);
}
}
pom.xml
XML
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.2.6</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>org.example</groupId>
<artifactId>config-server</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>config-server</name>
<description>config-server</description>
<properties>
<java.version>17</java.version>
<spring-cloud.version>2023.0.1</spring-cloud.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-config-server</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
Step 4: Run the Application
Once the Spring project is completed and successfully runs as a Spring application, it will start at port 8888.
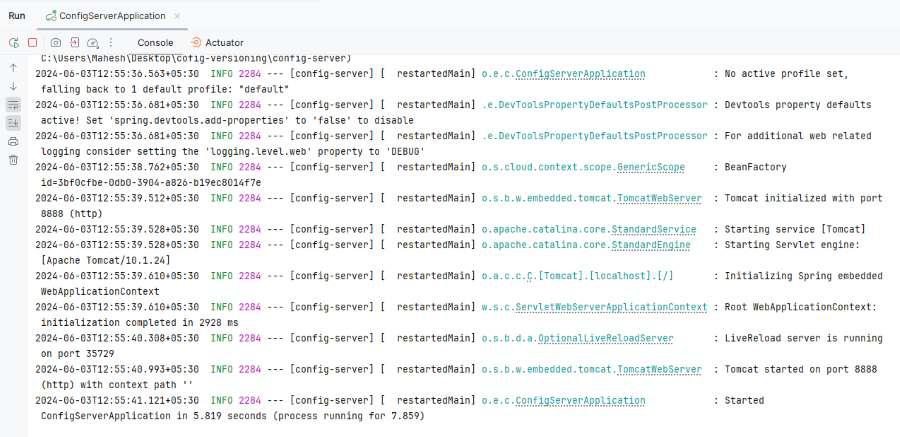
Create the Config-Client
Step 1: Create the spring project using spring initializer and add the below dependencies.
Dependencies:
- Spring Web
- Spring Dev Tools
- Lombok
- Spring Cloud Config Client
After creating the Spring project, the file structure will be like below:
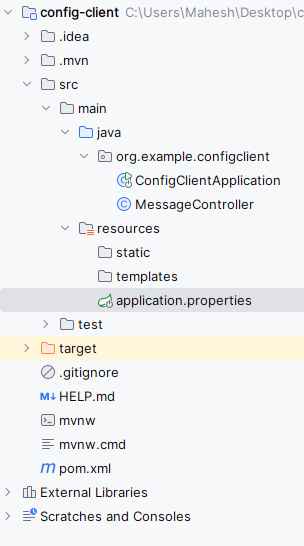
Step 2: Configure the Application properties
Open the application.properties file and add the configuring the server port and import git uri configuration
spring.application.name=config-client
spring.cloud.config.uri=http://localhost:8888
Step 3: Create the MesaageController class
Go to src > main > java > org.example.configclient > MessageController and put the below code.
Java
package org.example.configclient;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MessageController {
@Value("${message}")
private String message;
@GetMapping("/message")
public String getMessage() {
return this.message;
}
}
Step 4: Main Class
Open the main class (No changes are required) and put the below code.
Java
package org.example.configclient;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ConfigClientApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigClientApplication.class, args);
}
}
pom.xml
XML
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.2.6</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>org.example</groupId>
<artifactId>config-client</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>config-client</name>
<description>config-client</description>
<properties>
<java.version>17</java.version>
<spring-cloud.version>2023.0.1</spring-cloud.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
Step 5: Run the application
After the project completed, we will run this as a Spring Boot Application and it will start at port 8080.
.jpg)
Testing the Endpoints
GET http://localhost:8080/message
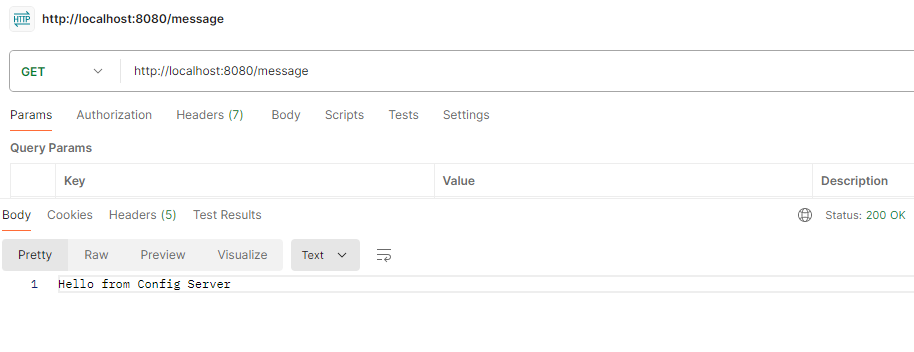
Update git application.properties file
Hello from Config Server - Version
commit and push the changes to Git repository
git add application.properties
git commit -m "Update configuration message to version 2"
git push
Again Endpoint Testing
GET http://localhost:8080/message
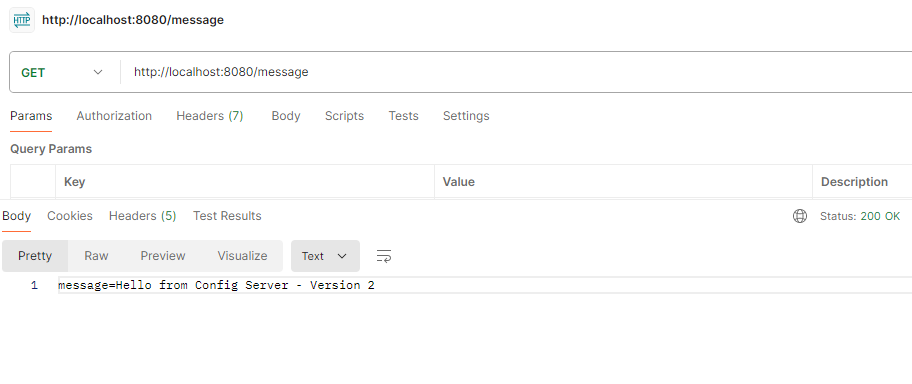
Conclusion
Implementing the configuration versioning with Spring Cloud Config allows you to manage the configuration centrally and leverage version control for the tracking changes of the application. This setup can enhances the maintainability and reliability of the microservices architecture. By the following the steps outlined above, we can easily set up and manage the configuration versioning in the Spring Cloud projects.
Please Login to comment...