Python Program to Reverse the Content of a File using Stack
Last Updated :
02 Aug, 2023
Given a file, the task is to change the content in reverse order using Stack, as well as store the lines of that file in reverse order in Python.
Examples:
Input:
1
2
3
4
5
Output:
5
4
3
2
1
Approach to Python Program to Reverse a Stack Using Recursion
- Create an empty stack.
- One by one push every line of the file to the stack.
- One by one pop each line from the stack and put them back to the file.
Input File:
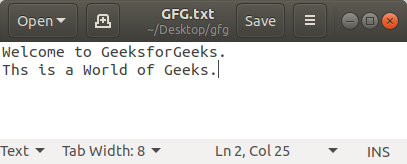
Code Explanation
Here first we create a stack class and then initialize an array in the class providing and provide the method to an array that follows the LIFO rule of the Python stack. The LIFO rule stands for Last Input Frist Output which is the basic rule of the stack after that we use that stack to store the data of the file and after storing the data we extract the data from the stack and print the data we append in the stack will come out first and like that we get the output data of the file reversed.
Python3
class Stack:
def __init__( self ):
self ._arr = []
def push( self , val):
self ._arr.append(val)
def is_empty( self ):
return len ( self ._arr) = = 0
def pop( self ):
if self .is_empty():
print ( "Stack is empty" )
return
return self ._arr.pop()
def reverse_file(filename):
S = Stack()
original = open (filename)
for line in original:
S.push(line.rstrip( "\n" ))
original.close()
output = open (filename, 'w' )
while not S.is_empty():
output.write(S.pop() + ";\n" )
output.close()
filename = "GFG.txt"
reverse_file(filename)
with open (filename) as file :
for f in file .readlines():
print (f, end = "")
|
Output:
This is a World of Geeks.
Welcome to GeeksforGeeks.
Time complexity: O(n), where n is the number of lines in the file.
Auxiliary space: O(n), where n is the number of lines in the file.
Please Login to comment...