Convert a Binary Tree into Doubly Linked List in spiral fashion
Last Updated :
30 Jun, 2022
Given a Binary Tree, convert it into a Doubly Linked List where the nodes are represented Spirally. The left pointer of the binary tree node should act as a previous node for created DLL and the right pointer should act as the next node.
The solution should not allocate extra memory for DLL nodes. It should use binary tree nodes for creating DLL i.e. only change of pointers is allowed.
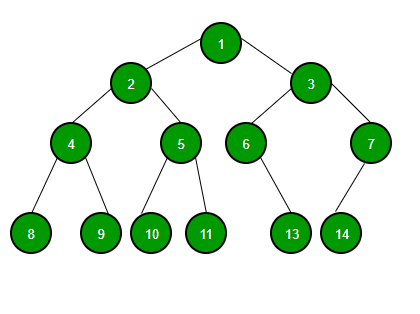
For example, for the tree on the left side, Doubly Linked List can be,
1 2 3 7 6 5 4 8 9 10 11 13 14 or
1 3 2 4 5 6 7 14 13 11 10 9 8.
We strongly recommend you minimize your browser and try this yourself first.
We can do this by doing a spiral order traversal in O(n) time and O(n) extra space. The idea is to use deque (Double-ended queue) that can be expanded or contracted on both ends (either its front or it’s back). We do something similar to level order traversal but to maintain spiral order, for every odd level, we dequeue node from the front and insert its left and right children in the back of the deque data structure. And for each even level, we dequeue node from the back and insert its right and left children in the front of the deque. We also maintain a stack to store Binary Tree nodes. Whenever we pop nodes from deque, we push that node into the stack.
Later, we pop all nodes from the stack and push the nodes at the beginning of the list. We can avoid the use of stack if we maintain a tail pointer that always points to the last node of DLL and inserts nodes in O(1) time in the end.
Below is the implementation of the above idea
C++
#include <bits/stdc++.h>
using namespace std;
struct Node
{
int data;
struct Node *left, *right;
};
void push(Node** head_ref, Node* node)
{
node->right = (*head_ref);
node->left = NULL;
if ((*head_ref) != NULL)
(*head_ref)->left = node ;
(*head_ref) = node;
}
void printList(Node *node)
{
while (node != NULL)
{
cout << node->data << " " ;
node = node->right;
}
}
void spiralLevelOrder(Node *root)
{
if (root == NULL)
return ;
deque<Node*> q;
q.push_front(root);
stack<Node*> stk;
int level = 0;
while (!q.empty())
{
int nodeCount = q.size();
if (level&1)
{
while (nodeCount > 0)
{
Node *node = q.front();
q.pop_front();
stk.push(node);
if (node->left != NULL)
q.push_back(node->left);
if (node->right != NULL)
q.push_back(node->right);
nodeCount--;
}
}
else
{
while (nodeCount > 0)
{
Node *node = q.back();
q.pop_back();
stk.push(node);
if (node->right != NULL)
q.push_front(node->right);
if (node->left != NULL)
q.push_front(node->left);
nodeCount--;
}
}
level++;
}
Node* head = NULL;
while (!stk.empty())
{
push(&head, stk.top());
stk.pop();
}
cout << "Created DLL is:\n" ;
printList(head);
}
Node* newNode( int data)
{
Node *temp = new Node;
temp->data = data;
temp->left = temp->right = NULL;
return temp;
}
int main()
{
Node *root = newNode(1);
root->left = newNode(2);
root->right = newNode(3);
root->left->left = newNode(4);
root->left->right = newNode(5);
root->right->left = newNode(6);
root->right->right = newNode(7);
root->left->left->left = newNode(8);
root->left->left->right = newNode(9);
root->left->right->left = newNode(10);
root->left->right->right = newNode(11);
root->right->left->right = newNode(13);
root->right->right->left = newNode(14);
spiralLevelOrder(root);
return 0;
}
|
Java
import java.util.*;
class Node
{
int data;
Node left, right;
public Node( int data)
{
this .data = data;
left = right = null ;
}
}
class BinaryTree
{
Node root;
Node head;
void push(Node node)
{
node.right = head;
node.left = null ;
if (head != null )
head.left = node;
head = node;
}
void printList(Node node)
{
while (node != null )
{
System.out.print(node.data + " " );
node = node.right;
}
}
void spiralLevelOrder(Node root)
{
if (root == null )
return ;
Deque<Node> q = new LinkedList<Node>();
q.addFirst(root);
Stack<Node> stk = new Stack<Node>();
int level = 0 ;
while (!q.isEmpty())
{
int nodeCount = q.size();
if ((level & 1 ) % 2 != 0 )
{
while (nodeCount > 0 )
{
Node node = q.peekFirst();
q.pollFirst();
stk.push(node);
if (node.left != null )
q.addLast(node.left);
if (node.right != null )
q.addLast(node.right);
nodeCount--;
}
}
else
{
while (nodeCount > 0 )
{
Node node = q.peekLast();
q.pollLast();
stk.push(node);
if (node.right != null )
q.addFirst(node.right);
if (node.left != null )
q.addFirst(node.left);
nodeCount--;
}
}
level++;
}
while (!stk.empty())
{
push(stk.peek());
stk.pop();
}
System.out.println( "Created DLL is : " );
printList(head);
}
public static void main(String[] args)
{
BinaryTree tree = new BinaryTree();
tree.root = new Node( 1 );
tree.root.left = new Node( 2 );
tree.root.right = new Node( 3 );
tree.root.left.left = new Node( 4 );
tree.root.left.right = new Node( 5 );
tree.root.right.left = new Node( 6 );
tree.root.right.right = new Node( 7 );
tree.root.left.left.left = new Node( 8 );
tree.root.left.left.right = new Node( 9 );
tree.root.left.right.left = new Node( 10 );
tree.root.left.right.right = new Node( 11 );
tree.root.right.left.right = new Node( 13 );
tree.root.right.right.left = new Node( 14 );
tree.spiralLevelOrder(tree.root);
}
}
|
Python3
class newNode:
def __init__( self , data):
self .data = data
self .left = None
self .right = None
def push(head_ref, node):
node.right = (head_ref)
node.left = None
if ((head_ref) ! = None ):
(head_ref).left = node
(head_ref) = node
def printList(node):
i = 0
while (i < len (node)):
print (node[i].data, end = " " )
i + = 1
def spiralLevelOrder(root):
if (root = = None ):
return
q = []
q.append(root)
stk = []
level = 0
while ( len (q)):
nodeCount = len (q)
if (level& 1 ):
while (nodeCount > 0 ):
node = q[ 0 ]
q.pop( 0 )
stk.append(node)
if (node.left ! = None ):
q.append(node.left)
if (node.right ! = None ):
q.append(node.right)
nodeCount - = 1
else :
while (nodeCount > 0 ):
node = q[ - 1 ]
q.pop( - 1 )
stk.append(node)
if (node.right ! = None ):
q.insert( 0 , node.right)
if (node.left ! = None ):
q.insert( 0 , node.left)
nodeCount - = 1
level + = 1
head = []
while ( len (stk)):
head.append(stk[ 0 ])
stk.pop( 0 )
print ( "Created DLL is:" )
printList(head)
if __name__ = = '__main__' :
root = newNode( 1 )
root.left = newNode( 2 )
root.right = newNode( 3 )
root.left.left = newNode( 4 )
root.left.right = newNode( 5 )
root.right.left = newNode( 6 )
root.right.right = newNode( 7 )
root.left.left.left = newNode( 8 )
root.left.left.right = newNode( 9 )
root.left.right.left = newNode( 10 )
root.left.right.right = newNode( 11 )
root.right.left.right = newNode( 13 )
root.right.right.left = newNode( 14 )
spiralLevelOrder(root)
|
C#
using System;
using System.Collections.Generic;
public class Node
{
public int data;
public Node left, right;
public Node( int data)
{
this .data = data;
left = right = null ;
}
}
public class BinaryTree
{
Node root;
Node head;
void push(Node node)
{
node.right = head;
node.left = null ;
if (head != null )
head.left = node;
head = node;
}
void printList(Node node)
{
while (node != null )
{
Console.Write(node.data + " " );
node = node.right;
}
}
void spiralLevelOrder(Node root)
{
if (root == null )
return ;
LinkedList<Node> q = new LinkedList<Node>();
q.AddFirst(root);
Stack<Node> stk = new Stack<Node>();
int level = 0;
while (q.Count != 0)
{
int nodeCount = q.Count;
if ((level & 1) % 2 != 0)
{
while (nodeCount > 0)
{
Node node = q.First.Value;
q.RemoveFirst();
stk.Push(node);
if (node.left != null )
q.AddLast(node.left);
if (node.right != null )
q.AddLast(node.right);
nodeCount--;
}
}
else
{
while (nodeCount > 0)
{
Node node = q.Last.Value;
q.RemoveLast();
stk.Push(node);
if (node.right != null )
q.AddFirst(node.right);
if (node.left != null )
q.AddFirst(node.left);
nodeCount--;
}
}
level++;
}
while (stk.Count != 0)
{
push(stk.Peek());
stk.Pop();
}
Console.WriteLine( "Created DLL is : " );
printList(head);
}
public static void Main(String[] args)
{
BinaryTree tree = new BinaryTree();
tree.root = new Node(1);
tree.root.left = new Node(2);
tree.root.right = new Node(3);
tree.root.left.left = new Node(4);
tree.root.left.right = new Node(5);
tree.root.right.left = new Node(6);
tree.root.right.right = new Node(7);
tree.root.left.left.left = new Node(8);
tree.root.left.left.right = new Node(9);
tree.root.left.right.left = new Node(10);
tree.root.left.right.right = new Node(11);
tree.root.right.left.right = new Node(13);
tree.root.right.right.left = new Node(14);
tree.spiralLevelOrder(tree.root);
}
}
|
Javascript
<script>
class Node
{
constructor(data)
{
this .data = data;
this .left = null ;
this .right = null ;
}
}
var root = null ;
var head = null ;
function push(node)
{
node.right = head;
node.left = null ;
if (head != null )
head.left = node;
head = node;
}
function printList(node)
{
while (node != null )
{
document.write(node.data + " " );
node = node.right;
}
}
function spiralLevelOrder(root)
{
if (root == null )
return ;
var q = [];
q.unshift(root);
var stk = [];
var level = 0;
while (q.length != 0)
{
var nodeCount = q.length;
if ((level & 1) % 2 != 0)
{
while (nodeCount > 0)
{
var node = q[0];
q.shift();
stk.push(node);
if (node.left != null )
q.push(node.left);
if (node.right != null )
q.push(node.right);
nodeCount--;
}
}
else
{
while (nodeCount > 0)
{
var node = q[q.length-1];
q.pop();
stk.push(node);
if (node.right != null )
q.unshift(node.right);
if (node.left != null )
q.unshift(node.left);
nodeCount--;
}
}
level++;
}
while (stk.length != 0)
{
push(stk[stk.length-1]);
stk.pop();
}
document.write( "Created DLL is :<br>" );
printList(head);
}
root = new Node(1);
root.left = new Node(2);
root.right = new Node(3);
root.left.left = new Node(4);
root.left.right = new Node(5);
root.right.left = new Node(6);
root.right.right = new Node(7);
root.left.left.left = new Node(8);
root.left.left.right = new Node(9);
root.left.right.left = new Node(10);
root.left.right.right = new Node(11);
root.right.left.right = new Node(13);
root.right.right.left = new Node(14);
spiralLevelOrder(root);
</script>
|
Output
Created DLL is:
1 2 3 7 6 5 4 8 9 10 11 13 14
Time Complexity: O(n), as we are using a loop to traverse n times. Where n is the number of nodes in the tree.
Auxiliary Space: O(n), as we are using extra space for dequeue and stack.
Please Login to comment...