jQuery Tutorial
Last Updated :
18 Jun, 2024
jQuery is a lightweight open-source JavaScript library that simplifies manipulating the Document Object Model (DOM), handling events, and creating dynamic web experiences. The main purpose of jQuery is to simplify the usage of JavaScript on websites. jQuery simplifies complex JavaScript tasks with single-line methods, it makes your code more readable and maintainable. By learning jQuery, you can significantly improve the interactivity and responsiveness of your web pages.
In this jQuery tutorial, you’ll learn all the basic to advanced concepts of jQuery such as DOM manipulation and event handling, AJAX, animations, plugins, etc.
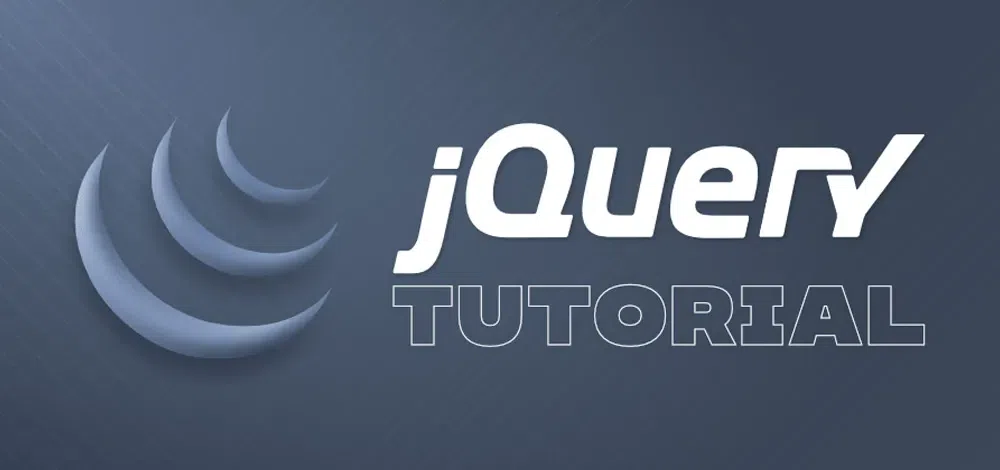
What is jQuery?
jQuery is a lightweight, “write less, do more” JavaScript library that simplifies web development. It provides an easy-to-use API for common tasks, making it much easier to work with JavaScript on your website. It streamlines web development by providing a concise syntax and powerful utilities for creating interactive and dynamic web pages.
Features of jQuery
Here are some key points about jQuery:
- DOM Manipulation: jQuery simplifies HTML DOM tree traversal and manipulation. You can easily select and modify elements on your web page.
- Event Handling: Handling user interactions (such as clicks or mouse events) becomes straightforward with jQuery.
- CSS Animations: You can create smooth animations and effects using jQuery.
- AJAX (Asynchronous JavaScript and XML): jQuery simplifies making asynchronous requests to the server and handling responses.
- Cross-Browser Compatibility: jQuery abstracts browser-specific inconsistencies, ensuring your code works consistently across different browsers.
- Community Support: A large community of developers actively contributes to jQuery, ensuring continuous updates and improvements.
- Plugins: jQuery has a wide range of plugins available for various tasks.
Getting Started with jQuery
1. Download the jQuery liberary:
You can download the jQuery library from the official website jquery.com and include it in your project by linking to it using a <script> tag, and host it on your server or local filesystem.
2. Include the jQuery Library:
1. Using jQuery Library Content Delivery Network (CDN) in your HTML project.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
2. Once the library is included, you can write jQuery code within <script>
tags. jQuery code typically follows a specific syntax:
<script> $(document).ready(function() { // Your jQuery code here });</script>
The $(document).ready(function() { ... })
function ensures your code executes only after the document is fully loaded, preventing errors.
Example:
In this example, we are using hover() and css() methods to change the style of heading content on mouse move over.
html
<!DOCTYPE html>
<html>
<head>
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js">
</script>
<script>
$(document).ready(function () {
$("h1").hover(
function () {
$(this).css(
"color",
"green"
);
},
function () {
$(this).css(
"color",
"aliceblue"
);
}
);
});
</script>
</head>
<body>
<h1>GeeksforGeeks</h1>
</body>
</html>
Output:
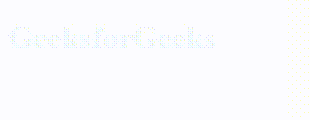
Learn More About jQuery Tutorial
jQuery Tutorial Complete References
jQuery Interview Questions and Answers (2024)
jQuery Selectors
jQuery offers a rich set of selectors to target specific elements on your webpage. Here are some commonly used ones:
- ID Selector: Select an element with a specific ID using
$("#element_id")
. - Class Selector: Target elements with a particular class using
$(".class_name")
. - Tag Selector: Select all elements of a specific tag using the tag name (e.g.,
"h1"
). - Attribute Selector: Target elements based on their attributes (e.g.,
$("input[type='text']")
selects all input elements with the type attribute set to “text”).
Essential jQuery Methods
html()
: Set or get the HTML content of an element.text()
: Set or get the text content of an element.attr()
: Get or set the value of an element’s attribute.addClass()
/removeClass()
/toggleClass()
: Add, remove, or toggle a class for an element.
Advantages of jQuery
- Wide range of plug-ins that allows developers to create plug-ins on top of the JavaScript library.
- Large development community.
- It is a lot easier to use compared to standard javascript and other javascript libraries.
- It lets users develop Ajax templates with ease. Ajax enables a sleeker interface where actions can be performed on pages without requiring the entire page to be reloaded.
- Being Light weight and a powerful chaining capabilities makes it more strong.
jQuery Cheat Sheet
The jQuery Cheat Sheet provides a quick reference guide for developers, summarizing common jQuery methods, selectors, events, and syntax, making it easier to write and understand jQuery code efficiently.
Please Login to comment...