Python input() Function
Last Updated :
12 Dec, 2022
Python input() function is used to take user input. By default, it returns the user input in form of a string.
input() Function
Syntax:
input(prompt)
prompt [optional]: any string value to display as input message
Ex: input(“What is your name? “)
Returns: Return a string value as input by the user.
By default input() function helps in taking user input as string.
If any user wants to take input as int or float, we just need to typecast it.
Refer to all datatypes and examples from here.
Python input() Function Example
Python3
color = input ( "What color is rose?: " )
print (color)
n = int ( input ( "How many roses?: " ))
print (n)
price = float ( input ( "Price of each rose?: " ))
print (price)
|
Output:
What color is rose?: red
red
How many roses?: 10
10
Price of each rose?: 15.50
15.5
Example 1: Taking the Name and Age of the user as input and printing it
By default, input returns a string. So the name and age will be stored as strings.
Python
name = input ( "Please Enter Your Name: " )
age = input ( "Please Enter Your Age: " )
print ( "Name & Age: " , name, age)
|
Output:
Please Enter Your Name: Rohit
Please Enter Your Age: 16
Name & Age: Rohit 16
Example 2: Taking two integers from users and adding them.
In this example, we will be looking at how to take integer input from users. To take integer input we will be using int() along with Python input()
Python
num1 = int ( input ( "Please Enter First Number: " ))
num2 = int ( input ( "Please Enter Second Number: " ))
addition = num1 + num2
print ( "The sum of the two given numbers is {} " . format (addition))
|
Output:
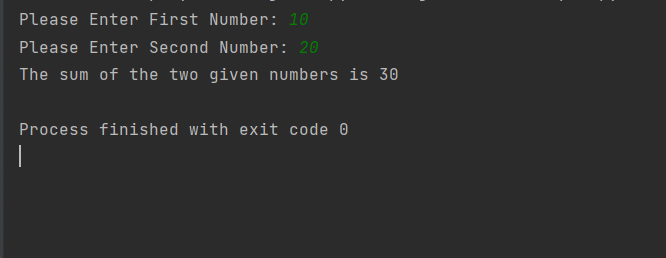
Similarly, we can use float() to take two float numbers. Let’s see one more example of how to take lists as input
Example 3: Taking Two lists as input and appending them
Taking user input as a string and splitting on each character using list() to convert into list of characters.
Python
list1 = list ( input ( "Please Enter Elements of list1: " ))
list2 = list ( input ( "Please Enter Elements of list2: " ))
for i in list2:
list1.append(i)
print (list1)
|
Output:
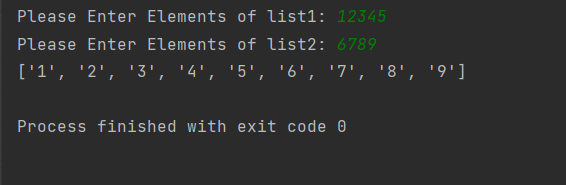
Please Login to comment...