In Python, list slicing is a common practice and it is the most used technique for programmers to solve efficient problems. Consider a Python list, in order to access a range of elements in a list, you need to slice a list. One way to do this is to use the simple slicing operator i.e. colon(:). With this operator, one can specify where to start the slicing, where to end, and specify the step. List slicing returns a new list from the existing list.
Python List Slicing Syntax
The format for list slicing is of Python List Slicing is as follows:
Lst[ Initial : End : IndexJump ]
If Lst is a list, then the above expression returns the portion of the list from index Initial to index End, at a step size IndexJump.
Indexing in Python List
Indexing is a technique for accessing the elements of a Python List. There are various ways by which we can access an element of a list.
Positive Indexes
In the case of Positive Indexing, the first element of the list has the index number 0, and the last element of the list has the index number N-1, where N is the total number of elements in the list (size of the list).
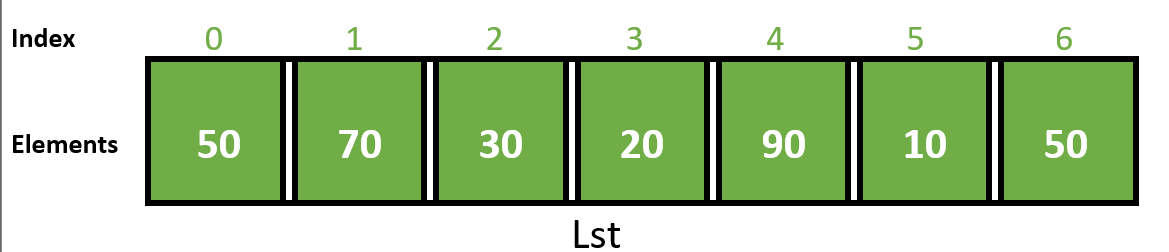
Positive Indexing of a Python List
Example:
In this example, we will display a whole list using positive index slicing.
Python
# Initialize list
Lst = [50, 70, 30, 20, 90, 10, 50]
# Display list
print(Lst[::])
Output:
[50, 70, 30, 20, 90, 10, 50]
Negative Indexes
The below diagram illustrates a list along with its negative indexes. Index -1 represents the last element and -N represents the first element of the list, where N is the length of the list.
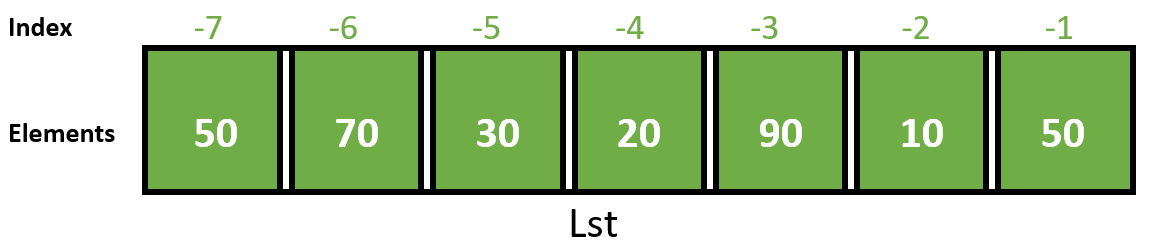
Negative Indexing of a Python List
Example:
In this example, we will access the elements of a list using negative indexing.
Python
# Initialize list
Lst = [50, 70, 30, 20, 90, 10, 50]
# Display list
print(Lst[-7::1])
Output:
[50, 70, 30, 20, 90, 10, 50]
Slicing
As mentioned earlier list slicing in Python is a common practice and can be used both with positive indexes as well as negative indexes. The below diagram illustrates the technique of list slicing:
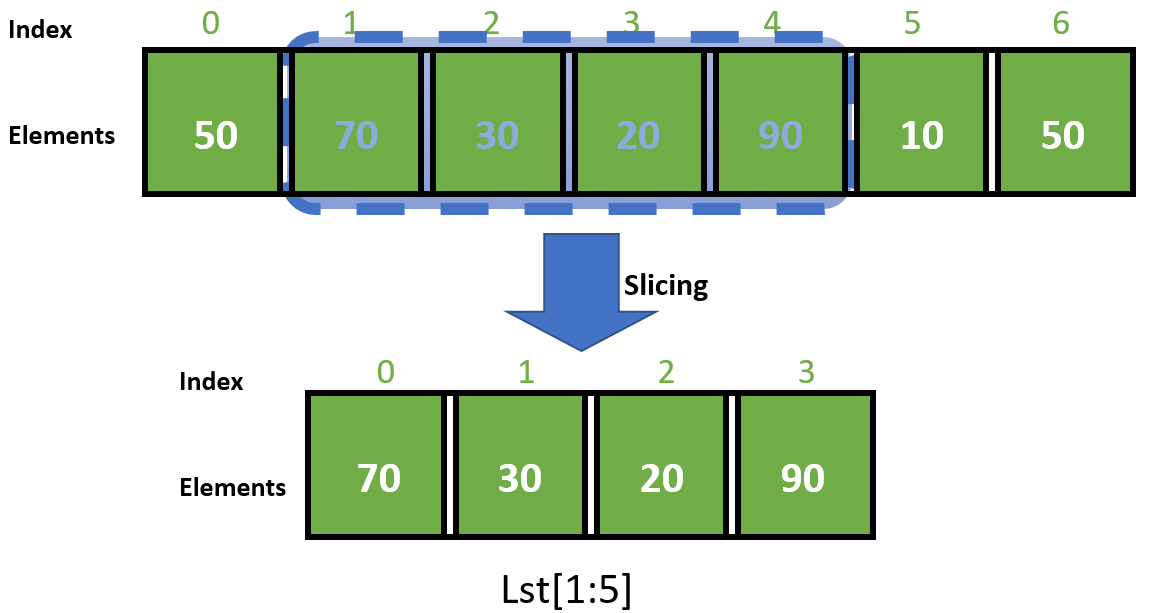
Python List Slicing
Example:
In this example, we will transform the above illustration into Python code.
Python
# Initialize list
Lst = [50, 70, 30, 20, 90, 10, 50]
# Display list
print(Lst[1:5])
Output:
[70, 30, 20, 90]
Examples of List Slicing in Python
Let us see some examples which depict the use of list slicing in Python.
Example 1: Leaving any argument like Initial, End, or IndexJump blank will lead to the use of default values i.e. 0 as Initial, length of the list as End, and 1 as IndexJump.
Python
# Initialize list
List = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Show original list
print("Original List:\n", List)
print("\nSliced Lists: ")
# Display sliced list
print(List[3:9:2])
# Display sliced list
print(List[::2])
# Display sliced list
print(List[::])
Output:
Original List:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
Sliced Lists:
[4, 6, 8]
[1, 3, 5, 7, 9]
[1, 2, 3, 4, 5, 6, 7, 8, 9]
Example 2: A reversed list can be generated by using a negative integer as the IndexJump argument. Leaving the Initial and End as blank. We need to choose the Initial and End values according to a reversed list if the IndexJump value is negative.Â
Python
# Initialize list
List = ['Geeks', 4, 'geeks !']
# Show original list
print("Original List:\n", List)
print("\nSliced Lists: ")
# Display sliced list
print(List[::-1])
# Display sliced list
print(List[::-3])
# Display sliced list
print(List[:1:-2])
Output:
Original List:
['Geeks', 4, 'geeks !']
Sliced Lists:
['geeks !', 4, 'Geeks']
['geeks !']
['geeks !']
Example 3: If some slicing expressions are made that do not make sense or are incomputable then empty lists are generated.
Python
# Initialize list
List = [-999, 'G4G', 1706256, '^_^', 3.1496]
# Show original list
print("Original List:\n", List)
print("\nSliced Lists: ")
# Display sliced list
print(List[10::2])
# Display sliced list
print(List[1:1:1])
# Display sliced list
print(List[-1:-1:-1])
# Display sliced list
print(List[:0:])
Output:
Original List:
[-999, 'G4G', 1706256, '^_^', 3.1496]
Sliced Lists:
[]
[]
[]
[]
Example 4: List slicing can be used to modify lists or even delete elements from a list.
Python
# Initialize list
List = [-999, 'G4G', 1706256, 3.1496, '^_^']
# Show original list
print("Original List:\n", List)
print("\nSliced Lists: ")
# Modified List
List[2:4] = ['Geeks', 'for', 'Geeks', '!']
# Display sliced list
print(List)
# Modified List
List[:6] = []
# Display sliced list
print(List)
Output:
Original List:
[-999, 'G4G', 1706256, 3.1496, '^_^']
Sliced Lists:
[-999, 'G4G', 'Geeks', 'for', 'Geeks', '!', '^_^']
['^_^']
Example 5: By concatenating sliced lists, a new list can be created or even a pre-existing list can be modified.Â
Python
# Initialize list
List = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Show original list
print("Original List:\n", List)
print("\nSliced Lists: ")
# Creating new List
newList = List[:3]+List[7:]
# Display sliced list
print(newList)
# Changing existing List
List = List[::2]+List[1::2]
# Display sliced list
print(List)
Output:
Original List:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
Sliced Lists:
[1, 2, 3, 8, 9]
[1, 3, 5, 7, 9, 2, 4, 6, 8]
Python List Slicing – FAQs
What does distinct() do in Python?
Python itself does not have a built-in distinct() function. However, the concept of “distinct” is commonly used in SQL databases to retrieve unique values. In Python, you can achieve the same effect using a set to filter out duplicate elements from an iterable.
How do you check if a list contains unique values in Python?
To check if a list contains only unique values, you can compare the length of the list with the length of the set created from the list:
def is_unique(lst):
return len(lst) == len(set(lst))
# Example usage
my_list = [1, 2, 3, 4, 5]
print(is_unique(my_list)) # Output: True
my_list_with_duplicates = [1, 2, 3, 3, 4, 5]
print(is_unique(my_list_with_duplicates)) # Output: False
What built-in Python data structure can be used to get unique values from a list?
The set data structure is used to get unique values from a list. A set automatically removes duplicates and maintains only unique elements.
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_values = list(set(my_list))
print(unique_values) # Output: [1, 2, 3, 4, 5]
How do I count unique items in a list in Python?
To count the number of unique items in a list, convert the list to a set and get the length of the set:
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_count = len(set(my_list))
print(unique_count) # Output: 5
How do I check if a list contains duplicates in Python?
To check if a list contains duplicates, you can compare the length of the list with the length of the set created from the list. If they are not equal, the list contains duplicates:
def contains_duplicates(lst):
return len(lst) != len(set(lst))
# Example usage
my_list = [1, 2, 3, 3, 4, 5]
print(contains_duplicates(my_list)) # Output: True
my_unique_list = [1, 2, 3, 4, 5]
print(contains_duplicates(my_unique_list)) # Output: False
Please Login to comment...