Index Mapping (or Trivial Hashing) with negatives allowed
Last Updated :
02 Jun, 2023
Index Mapping (also known as Trivial Hashing) is a simple form of hashing where the data is directly mapped to an index in a hash table. The hash function used in this method is typically the identity function, which maps the input data to itself. In this case, the key of the data is used as the index in the hash table, and the value is stored at that index.
For example, if we have a hash table of size 10 and we want to store the value “apple” with the key “a”, the trivial hashing function would simply map the key “a” to the index “a” in the hash table, and store the value “apple” at that index.
One of the main advantages of Index Mapping is its simplicity. The hash function is easy to understand and implement, and the data can be easily retrieved using the key. However, it also has some limitations. The main disadvantage is that it can only be used for small data sets, as the size of the hash table has to be the same as the number of keys. Additionally, it doesn’t handle collisions, so if two keys map to the same index, one of the data will be overwritten.
Given a limited range array contains both positive and non-positive numbers, i.e., elements are in the range from -MAX to +MAX. Our task is to search if some number is present in the array or not in O(1) time.
Since the range is limited, we can use index mapping (or trivial hashing). We use values as the index in a big array. Therefore we can search and insert elements in O(1) time.
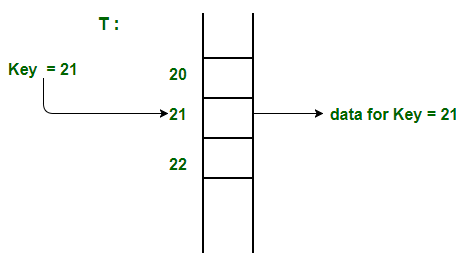
How to handle negative numbers?
The idea is to use a 2D array of size hash[MAX+1][2]
Algorithm:
Assign all the values of the hash matrix as 0.
Traverse the given array:
- If the element ele is non negative assign
- Else take the absolute value of ele and
- assign hash[ele][1] as 1.
To search any element x in the array.
- If X is non-negative check if hash[X][0] is 1 or not. If hash[X][0] is one then the number is present else not present.
- If X is negative take the absolute value of X and then check if hash[X][1] is 1 or not. If hash[X][1] is one then the number is present
Below is the implementation of the above idea.
C++
#include <bits/stdc++.h>
using namespace std;
#define MAX 1000
bool has[MAX + 1][2];
bool search( int X)
{
if (X >= 0) {
if (has[X][0] == 1)
return true ;
else
return false ;
}
X = abs (X);
if (has[X][1] == 1)
return true ;
return false ;
}
void insert( int a[], int n)
{
for ( int i = 0; i < n; i++) {
if (a[i] >= 0)
has[a[i]][0] = 1;
else
has[ abs (a[i])][1] = 1;
}
}
int main()
{
int a[] = { -1, 9, -5, -8, -5, -2 };
int n = sizeof (a)/ sizeof (a[0]);
insert(a, n);
int X = -5;
if (search(X) == true )
cout << "Present" ;
else
cout << "Not Present" ;
return 0;
}
|
Java
class GFG
{
final static int MAX = 1000 ;
static boolean [][] has = new boolean [MAX + 1 ][ 2 ];
static boolean search( int X)
{
if (X >= 0 )
{
if (has[X][ 0 ] == true )
{
return true ;
}
else
{
return false ;
}
}
X = Math.abs(X);
if (has[X][ 1 ] == true )
{
return true ;
}
return false ;
}
static void insert( int a[], int n)
{
for ( int i = 0 ; i < n; i++)
{
if (a[i] >= 0 )
{
has[a[i]][ 0 ] = true ;
}
else
{
int abs_i = Math.Abs(a[i]);
has[abs_i][ 1 ] = true ;
}
}
}
public static void main(String args[])
{
int a[] = {- 1 , 9 , - 5 , - 8 , - 5 , - 2 };
int n = a.length;
insert(a, n);
int X = - 5 ;
if (search(X) == true )
{
System.out.println( "Present" );
}
else
{
System.out.println( "Not Present" );
}
}
}
|
Python3
def search(X):
if X > = 0 :
return has[X][ 0 ] = = 1
X = abs (X)
return has[X][ 1 ] = = 1
def insert(a, n):
for i in range ( 0 , n):
if a[i] > = 0 :
has[a[i]][ 0 ] = 1
else :
has[ abs (a[i])][ 1 ] = 1
if __name__ = = "__main__" :
a = [ - 1 , 9 , - 5 , - 8 , - 5 , - 2 ]
n = len (a)
MAX = 1000
has = [[ 0 for i in range ( 2 )]
for j in range ( MAX + 1 )]
insert(a, n)
X = - 5
if search(X) = = True :
print ( "Present" )
else :
print ( "Not Present" )
|
C#
Javascript
<script>
let MAX = 1000;
let has = new Array(MAX+1);
for (let i=0;i<MAX+1;i++)
{
has[i]= new Array(2);
for (let j=0;j<2;j++)
has[i][j]=0;
}
function search(X)
{
if (X >= 0)
{
if (has[X][0] == true )
{
return true ;
}
else
{
return false ;
}
}
X = Math.abs(X);
if (has[X][1] == true )
{
return true ;
}
return false ;
}
function insert(a,n)
{
for (let i = 0; i < n; i++)
{
if (a[i] >= 0)
{
has[a[i]][0] = true ;
}
else
{
let abs_i = Math.abs(a[i]);
has[abs_i][1] = true ;
}
}
}
let a=[-1, 9, -5, -8, -5, -2];
let n = a.length;
insert(a, n);
let X = -5;
if (search(X) == true )
{
document.write( "Present" );
}
else
{
document.write( "Not Present" );
}
</script>
|
Time Complexity: The time complexity of the above algorithm is O(N), where N is the size of the given array.
Space Complexity: The space complexity of the above algorithm is O(N), because we are using an array of max size.
Please Login to comment...