Python Program for KMP Algorithm for Pattern Searching
Last Updated :
08 Jun, 2022
Given a text txt[0..n-1] and a pattern pat[0..m-1], write a function search(char pat[], char txt[]) that prints all occurrences of pat[] in txt[]. You may assume that n > m.
Examples:
Input: txt[] = "THIS IS A TEST TEXT"
pat[] = "TEST"
Output: Pattern found at index 10
Input: txt[] = "AABAACAADAABAABA"
pat[] = "AABA"
Output: Pattern found at index 0
Pattern found at index 9
Pattern found at index 12
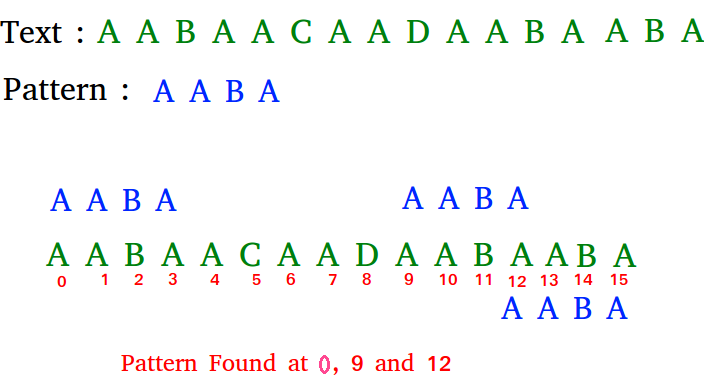
Pattern searching is an important problem in computer science. When we do search for a string in notepad/word file or browser or database, pattern searching algorithms are used to show the search results.
Python3
def KMPSearch(pat, txt):
M = len (pat)
N = len (txt)
lps = [ 0 ] * M
j = 0
computeLPSArray(pat, M, lps)
i = 0
while i < N:
if pat[j] = = txt[i]:
i + = 1
j + = 1
if j = = M:
print ( "Found pattern at index" , str (i - j))
j = lps[j - 1 ]
elif i < N and pat[j] ! = txt[i]:
if j ! = 0 :
j = lps[j - 1 ]
else :
i + = 1
def computeLPSArray(pat, M, lps):
len = 0
lps[ 0 ]
i = 1
while i < M:
if pat[i] = = pat[ len ]:
len + = 1
lps[i] = len
i + = 1
else :
if len ! = 0 :
len = lps[ len - 1 ]
else :
lps[i] = 0
i + = 1
txt = "ABABDABACDABABCABAB"
pat = "ABABCABAB"
KMPSearch(pat, txt)
|
Output:
Found pattern at index 10
Time Complexity: O(m+n)
Space Complexity: O(m)
Please refer complete article on KMP Algorithm for Pattern Searching for more details!
Please Login to comment...