Python – Write dictionary of list to CSV
Last Updated :
12 Jul, 2022
In this article, we will discuss the practical implementation of how to write a dictionary of lists to CSV.
We can use the csv module for this. The csvwriter file object supports three methods such as csvwriter.writerow(), csvwriter.writerows(), csvwriter.writeheader().
Syntax:
csv.writer(csvfile, dialect='excel')
Parameters:
- csvfile: A file object with write() method.
- dialect (optional): Name of the dialect to be used.
To write a dictionary of list to CSV files, the necessary functions are csv.writer(), csv.writerow(). This method writes a single row at a time. Field rows can be written using this method.
Syntax :
csvwriter.writerow(row)
Example 1:
Python3
import csv
with open ( 'test.csv' , 'w' ) as testfile:
fieldnames = [ 'first_field' , 'second_field' , 'third_field' ]
writer = csv.DictWriter(testfile, fieldnames = fieldnames)
writer.writeheader()
|
Output:
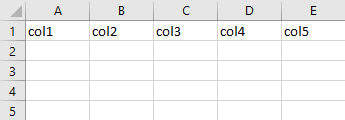
Alternatively, a dictionary of lists can be converted to a CSV file using only the csv.writerow() function, just by iterating through each key-value pair in the dictionary as shown
Example 2:
Python3
test = { 'Age' : [ 52 , 24 , 31 , 47 , 51 , 61 ],
'Sex' : [ 'F' , 'M' , 'M' , 'F' , 'F' , 'M' ],
'height' : [ 143 , 163 , 144 , 154 , 174 , 177 ],
'weight' : [ 77 , 66 , 59 , 53 , 71 , 63 ], }
with open ( "test.csv" , "w" ) as outfile:
writer = csv.writer(outfile)
key_list = list (test.keys())
limit = len (key_list)
writer.writerow(test.keys())
for i in range (limit):
writer.writerow([test[x][i] for x in key_list])
|
Output:
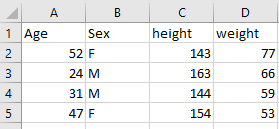
To write a dictionary of list to CSV files, the necessary functions are csv.writer(), csv.writerows(). This method writes all elements in rows (an iterable of row objects as described above) to the writer’s file object.
Syntax
csvwriter.writerows(rows)
Example 1:
Python3
import csv
with open ( 'test.csv' , 'w' ) as testfile:
csvwriter = csv.writer(testfile)
csvwriter.writerows([[ 'row1' ], [ 'row2' ], [ 'row3' ],
[ 'row4' ], [ 'row5' ], [ 'row6' ]])
|
Output:
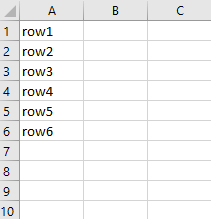
Example 2:
Here we are going to create a csv file test.csv and store it in a variable as outfile.
Python3
test = { 'Age' : [ 52 , 24 , 31 , 47 , 51 , 61 ],
'Sex' : [ 'F' , 'M' , 'M' , 'F' , 'F' , 'M' ],
'height' : [ 143 , 163 , 144 , 154 , 174 , 177 ],
'weight' : [ 77 , 66 , 59 , 53 , 71 , 63 ], }
with open ( "test.csv" , "w" ) as outfile:
writer = csv.writer(outfile)
writer.writerow(test.keys())
writer.writerows( zip ( * test.values()))
|
Output:
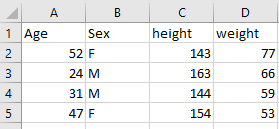
This method writes a row with the field names specified, as header to the csv.dictwriter object.
Syntax
csvwriter.writeheader()
Example:
Python3
import csv
with open ( 'test.csv' , 'w' ) as testfile:
fieldnames = [ 'first_field' , 'second_field' , 'third_field' ]
writer = csv.DictWriter(testfile, fieldnames = fieldnames)
writer.writeheader()
|
Output:
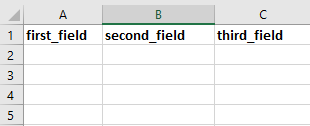
Please Login to comment...