How to add timestamp to CSV file in Python
Last Updated :
23 Aug, 2021
Prerequisite: Datetime module
In this example, we will learn How to add timestamp to CSV files in Python. We can easily add timestamp to CSV files with the help of datetime module of python. Let’s the stepwise implementation for adding timestamp to CSV files in Python.
Creating CSV and adding timestamp
- Import csv and datetime module. We will use the csv module to read and write the csv file and datetime module to add the current date and time in the csv file
- Take the data from the user.
- Open the CSV file in read and write mode (‘r+’) using open() function.
- The open() function opens a file and returns its as a file-object.
- newline = ‘ ‘ controls how universal newlines mode works. It can be None, ‘ ‘, ‘\n’, ‘\r’, and ‘\r\n’.
- write() returns a writer object which is responsible for converting the user’s data into a delimited string.
- Get current date and time using the datetime.now() function of datetime module.
- Iterate over all the data in the rows variable with the help of a for loop.
- Insert the current date and time at 0th index in every data using the insert() function.
- Write the data using writerow() in the CSV file with the current date and time.
Example 1: Add timestamp to CSV file
Python3
import csv
from datetime import datetime
rows = [[ 'GeeksforGeeks1' , 'GeeksforGeeks2' ],
[ 'GeeksforGeeks3' , 'GeeksforGeeks4' ],
[ 'GeeksforGeeks5' , 'GeeksforGeeks6' ]]
with open (r 'YOUR_CSV_FILE.csv' , 'r+' , newline = '') as file :
file_write = csv.writer( file )
current_date_time = datetime.now()
for val in rows:
val.insert( 0 , current_date_time)
file_write.writerow(val)
|
Output :
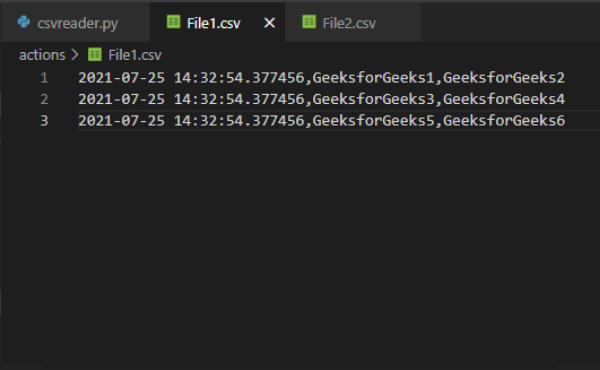
Example 2: Adding timestamp to CSV file
Python3
import csv
from datetime import datetime
def write_in_csv(rows):
with open (r 'YOUR_CSV_FILE.csv' , 'r+' , newline = '') as file :
file_write = csv.writer( file )
for val in rows:
file_write.writerow(val)
rows = []
run = ''
while run ! = 'no' :
val = []
val1 = input ( "Enter 1st value:- " )
val2 = input ( "Enter 2nd value:- " )
val3 = input ( "Enter 3rd value:- " )
current_date_time = datetime.now()
val.append(current_date_time)
val.append(val1)
val.append(val2)
val.append(val3)
run = input ( "Do you want to add one more row? Type Yes or No:- " )
run = run.lower()
rows.append(val)
write_in_csv(rows)
|
Output:
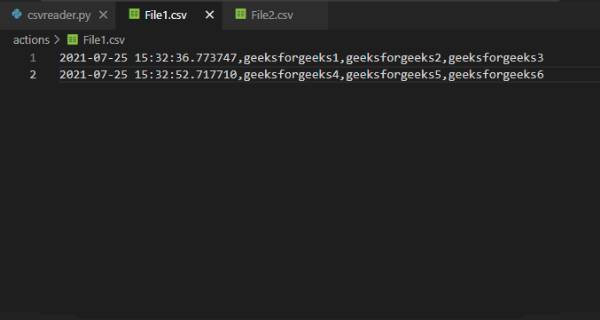
Adding Timestamps in Existing CSV file
It is also possible to add timestamp to a CSV file that already contains some data. For this open the first file in read mode and the second file in write mode. Creating a csv reader object of the first file using the reader() function of csv module. reader() return a reader object which will iterate over lines in the given CSV file.
Append every data stored in the first file in rows variable using a for loop. Create a writer object of the second file using the writer() function of csv module. Now iterate over all the data in the rows variable using a for loop. Store the current date and time in a variable and then inserting it in the data at 0th index using the insert() function. Write the stored data in File2 using the writerow() function of csv module.
Example 1: Adding timestamp to existing data
Content of File1:
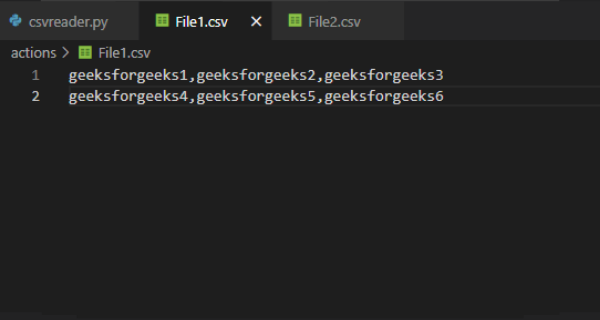
Python3
import csv
from datetime import datetime
rows = []
with open (r 'FILE1.csv' , 'r' , newline = '') as file :
with open (r 'FILE2.csv' , 'w' , newline = '') as file2:
reader = csv.reader( file , delimiter = ',' )
for row in reader:
rows.append(row)
file_write = csv.writer(file2)
for val in rows:
current_date_time = datetime.now()
val.insert( 0 , current_date_time)
file_write.writerow(val)
|
Output:
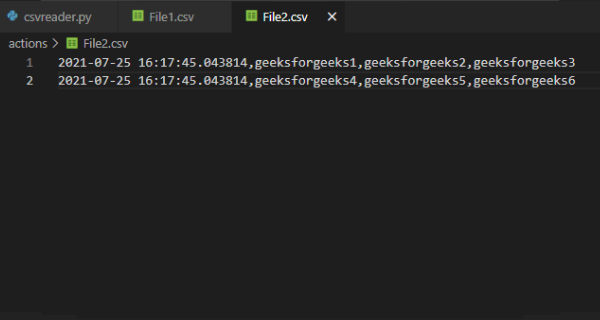
Please Login to comment...