Sorting a CSV object by dates in Python
Last Updated :
25 Feb, 2021
CSV stands for comma-separated values. A CSV file can be opened in Google Sheets or Excel and will be formatted as a spreadsheet. However, a CSV file is actually a plain-text file. It can also be opened with a text editor program such as Atom. In this article, we are going to see how to sort a CSV object by dates in Python
CSVs give us a good, simple way to organize data without using a database program. It’s easy to read from and write to CSV files with Python.
Steps to Read a CSV file
Step 1: In the first step to read a CSV you need to find the file.
Step 2: Import pandas library
The pandas library is exported by using import keyword and object as pd which is a standard notation for pandas library.
Step 3: Read the CSV file by using pandas library and assign it to a variable.
The csv file ‘data.csv’ is loaded by using read_csv method present in the pandas library and stored in the variable named ‘data’ and now this variable is called a dataframe.
Python3
data = pd.read_csv( 'data.csv' )
|
Step 4: Display the first 5 rows of the data by using head function.
The ‘.head()’ method is used to print the first 5 rows and ‘.tail()’ method is used to print the last 5 rows of the data file.
Output:
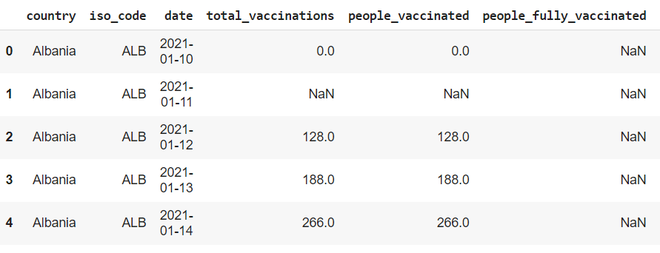
output screenshot
Steps to sort the Data by Dates
Step 1: Convert the Date column into required date time format
You can use the parameter infer_datetime_format. Example with your sample data below:
Python3
data[ 'date' ] = pd.to_datetime(data.date, infer_datetime_format = True )
display(data.head())
|
Output:
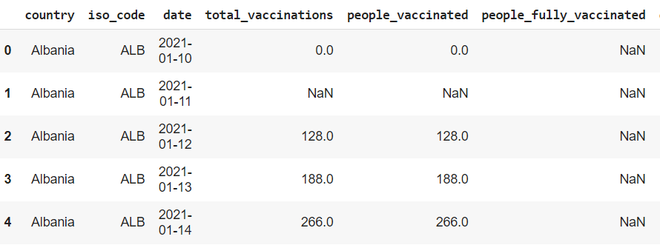
output screenshot
Step 2: Use the sort_values method and giving parameter as date we sort the values by date. To get the sorted while use head function to get first 5 rows of dataInput:
Python3
data.sort_values(by = 'date' , ascending = True , inplace = True )
display(data.head())
|
Output:
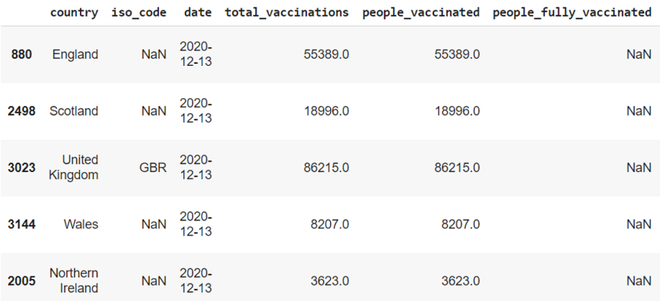
Please Login to comment...