Convert CSV to JSON using Python
Last Updated :
21 Jan, 2021
CSV (or Comma Separated Value) files represent data in a tabular format, with several rows and columns. An example of a CSV file can be an Excel Spreadsheet. These files have the extension of .csv, for instance, geeksforgeeks.csv. In this sample file, every row will represent a record of the dataset, and each column will indicate a unique feature variable.
On the other hand, JSON (or JavaScript Object Notation) is a dictionary-like notation that can be used by importing the JSON package in Python. Every record (or row) is saved as a separate dictionary, with the column names as Keys of the dictionary. All of these records as dictionaries are saved in a nested dictionary to compose the entire dataset. It is stored with the extension .json, for example, geeksforgeeks.json
Refer to the below articles to understand the basics of JSON and CSV.
Converting CSV to JSON
We will create a JSON file that will have several dictionaries, each representing a record (row) from the CSV file, with the Key as the column specified.
Sample CSV File used:
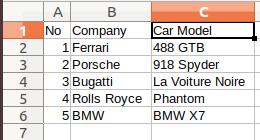
Python3
import csv
import json
def make_json(csvFilePath, jsonFilePath):
data = {}
with open (csvFilePath, encoding = 'utf-8' ) as csvf:
csvReader = csv.DictReader(csvf)
for rows in csvReader:
key = rows[ 'No' ]
data[key] = rows
with open (jsonFilePath, 'w' , encoding = 'utf-8' ) as jsonf:
jsonf.write(json.dumps(data, indent = 4 ))
csvFilePath = r 'Names.csv'
jsonFilePath = r 'Names.json'
make_json(csvFilePath, jsonFilePath)
|
Output:

Please Login to comment...