Convert JSON to dictionary in Python
Last Updated :
20 Jun, 2024
JSON stands for JavaScript Object Notation. It means that a script (executable) file which is made of text in a programming language, is used to store and transfer the data. Python supports JSON through a built-in package called JSON. To use this feature, we import the Python JSON package into Python script. The text in JSON is done through quoted-string which contains a value in key-value mapping within { }. It is similar to the dictionary in Python.
Function Used
json.load(): json.load() function is present in Python built-in ‘JSON’ module. This function is used to parse the JSON string.
json.loads(): json.loads() function is present in Python built-in ‘json’ module. This function is used to parse the JSON string.
Convert JSON String to Dictionary Python
In this example, we are going to convert a JSON string to Python Dictionary using json.loads() method of JSON module in Python. Firstly, we import json module and then define JSON string after that converting JSON string to Python dictionary by passing it to json.loads() in parameter. We have print the dictionary and their values using the keys as seen in the output.
Python
# Import JSON module
import json
# Define JSON string
jsonString = '{ "id": 121, "name": "Naveen", "course": "MERN Stack"}'
# Convert JSON String to Python
student_details = json.loads(jsonString)
# Print Dictionary
print(student_details)
# Print values using keys
print(student_details['name'])
print(student_details['course'])
Output{'id': 121, 'name': 'Naveen', 'course': 'MERN Stack'}
Naveen
MERN Stack
Convert JSON File to Python Object
Below is the JSON file that we will convert to Python dictionary using json.load() mehtod.
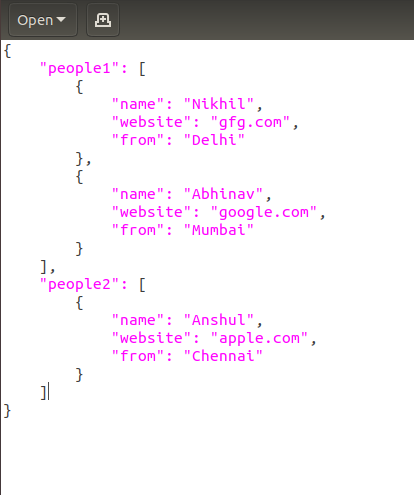
In the below code, firstly we open the “data.json” file using file handling in Python and then convert the file to Python object using the json.load() method we have also print the type of data after conversion and print the dictionary.
Python
# Python program to demonstrate
# Conversion of JSON data to
# dictionary
# importing the module
import json
# Opening JSON file
with open('data.json') as json_file:
data = json.load(json_file)
# Print the type of data variable
print("Type:", type(data))
# Print the data of dictionary
print("\nPeople1:", data['people1'])
print("\nPeople2:", data['people2'])
Output :

Convert Nested JSON Object to Dictionary
In this example, we will convert the nested JSON into a Python dictionary. For JSON data we will use the same JSON file used in the above example.
Python
# importing the module
import json
# Opening JSON file
with open('data.json') as json_file:
data = json.load(json_file)
# for reading nested data [0] represents
# the index value of the list
print(data['people1'][0])
# for printing the key-value pair of
# nested dictionary for loop can be used
print("\nPrinting nested dictionary as a key-value pair\n")
for i in data['people1']:
print("Name:", i['name'])
print("Website:", i['website'])
print("From:", i['from'])
print()
Output :
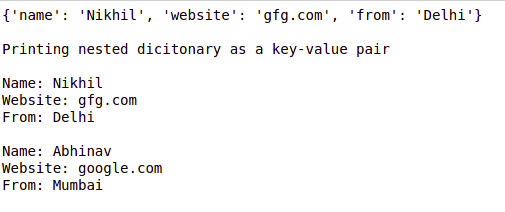
Convert JSON String to Dictionary in Python
In this example, we will convert the json string into Python dictionary using json.loads() method. Firstly, we will import JSON module. Create a json string and store it in a variable ‘json_string’ after that we will convert the json string into dictionary by passing ‘json_string’ into json.loads() as argument and store the converted dictionary in ‘json_dict’. Finally, print the Python dictionary.
Python
import json
# JSON string
json_string = '{"Name": "Suezen", "age": 23, "Course": "DSA"}'
# Convert JSON string to dictionary
json_dict = json.loads(json_string)
print(json_dict)
Output{'Name': 'Suezen', 'age': 23, 'Course': 'DSA'}
Convert JSON to dictionary in Python – FAQs
How to parse JSON into Python object?
You can parse JSON data into a Python object (typically a dictionary or list) using the json.loads()
function.
import json
json_str = '{"name": "John", "age": 30, "city": "New York"}'
python_obj = json.loads(json_str)
print(python_obj)
# Output: {'name': 'John', 'age': 30, 'city': 'New York'}
How to convert JSON text to dictionary in Python?
JSON text can be converted to a dictionary using the json.loads()
function as shown in the previous example.
import json
json_text = '{"name": "Alice", "age": 25}'
dictionary = json.loads(json_text)
print(dictionary)
# Output: {'name': 'Alice', 'age': 25}
How to extract JSON data in Python?
To extract data from a JSON string, parse it into a Python object (dictionary or list) using json.loads()
and then access the specific data using standard dictionary or list operations.
import json
json_data = '{"name": "Bob", "age": 35, "city": "Chicago"}'
data = json.loads(json_data)
name = data['name']
age = data['age']
print(f"Name: {name}, Age: {age}")
# Output: Name: Bob, Age: 35
How to convert JSON to readable format in Python?
To convert JSON data to a more readable format in Python, you can use the json.dumps()
function with the indent
parameter.
import json
json_data = '{"name": "Eve", "age": 28, "city": "Seattle"}'
python_obj = json.loads(json_data)
formatted_json = json.dumps(python_obj, indent=4)
print(formatted_json)
This will produce:
{
"name": "Eve",
"age": 28,
"city": "Seattle"
}
How to convert JSON string to dictionary in Python?
You can convert a JSON string directly into a dictionary using json.loads()
.
import json
json_str = '{"key1": "value1", "key2": "value2"}'
dictionary = json.loads(json_str)
print(dictionary)
# Output: {'key1': 'value1', 'key2': 'value2'}
Please Login to comment...