How to check which Button was clicked in Tkinter ?
Last Updated :
25 Jan, 2022
Are you using various buttons in your app, and you are being confused about which button is being pressed? Don’t know how to get rid of this solution!! Don’t worry, just go through the article. In this article, we will explain in detail the procedure to know which button was pressed.
Steps by Step Approach:
Step 1: First, import the library Tkinter.
from tkinter import *
Step 2: Now, create a GUI app using Tkinter.
app = Tk()
Step 3: Then, create a function with one parameter, i.e., of the text you want to show when a button is clicked
def which_button(button_press):
print (button_press)
Step 4: Further, create and display the first button by calling the which_button function you declared in step 3.
b1 = Button(app, text="#Text you want to show in button b1",
command=lambda m="#Text you want to show when\
b1 is clicked": which_button(m))
b1.grid(padx=10, pady=10)
Step 5: Moreover, create and display the second button by calling the which_button function you declared in step 3.
b2 = Button(app, text="#Text you want to show in button b2",
command=lambda m="#Text you want to show when \
b2 is clicked": which_button(m))
b2.grid(padx=10, pady=10)
Step 6: Next, keep on repeating steps 4 and 5 for n number of buttons by replacing n with the number of buttons you want it to display on the app. Don’t forget to call the which_button function you declared in step 3.
bn = Button(app, text="#Text you want to show in button bn",
command=lambda m="#Text you want to show when \
bn is clicked": which_button(m))
bn.grid(padx=10, pady=10)
Step 7: Finally, create an infinite loop for displaying the app on the screen.
app.mainloop()
Example:
In this example, if the text ‘It is an apple‘ is printed on the screen, we get to know ‘Apple‘ button is pressed, else when ‘It is a banana‘ is printed on the screen, we get to know ‘Banana‘ button is pressed.
Python
from tkinter import *
app = Tk()
def which_button(button_press):
print (button_press)
b1 = Button(app, text = "Apple" ,
command = lambda m = "It is an apple" : which_button(m))
b1.grid(padx = 10 , pady = 10 )
b2 = Button(app, text = "Banana" ,
command = lambda m = "It is a banana" : which_button(m))
b2.grid(padx = 10 , pady = 10 )
app.mainloop()
|
Output:
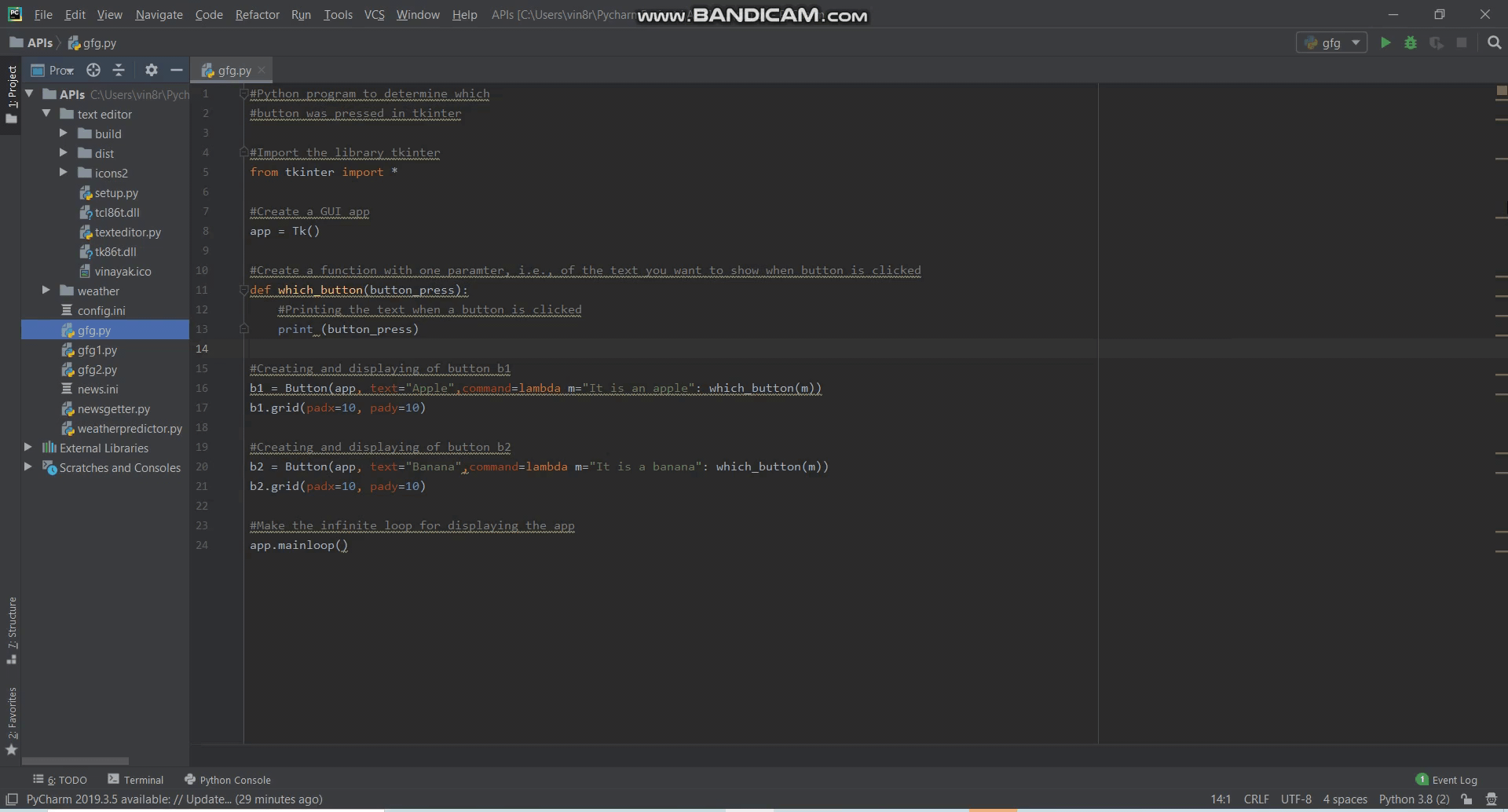
Please Login to comment...