How to Change Tkinter LableFrame Border Color?
Last Updated :
01 Apr, 2021
LableFrame in Tkinter is the widget that creates the rectangular area which contains other widgets. In this article, we are going to see how we can change the border of the label frame. But for achieving the color, we need to go through the theme for Tkinter, hence we use ttk module for the same which is inbuilt in python 3 Tkinter.
Step by Step Implementation:
Step 1: Importing all the required modules
Python3
import tkinter as tk
import tkinter.ttk as ttk
|
Step 2: Building the label frame and put some widgets inside it.
Python3
import tkinter as tk
import tkinter.ttk as ttk
root = tk.Tk()
root.geometry( "300x300" )
labelframe = ttk.LabelFrame(root, text = "GFG" )
labelframe.pack(padx = 30 , pady = 30 )
left = tk.Label(labelframe, text = "Geeks for Geeks" )
left.pack()
root.mainloop()
|
Output:
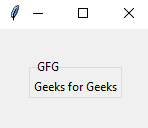
Example 1: Using Clam theme in Tkinter.
Clam is a theme for tkinter windows and widgets. It comes under ttk module. The syntax for creating clam ttk window:
style = ttk.Style()
style.theme_use('clam')
Note: We use a clam theme in ttk module which enables using properties like border colors. Other themes may not have properties like border color.
Below is the implementation:
Python3
import tkinter as tk
import tkinter.ttk as ttk
root = tk.Tk()
style = ttk.Style()
style.theme_use( 'clam' )
style.configure( "TLabelframe" , bordercolor = "red" )
labelframe = ttk.LabelFrame(root, text = "GFG" )
labelframe.grid(padx = 30 , pady = 30 )
left = tk.Label(labelframe, text = "Geeks for Geeks" )
left.pack()
root.mainloop()
|
Output:
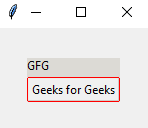
Example 2: Building our own style functionality theme.
Syntax:
# creating the theme
style.theme_create(‘class_name’, # Name of the class
settings={
‘TLabelframe’: { # Name of the widget
‘configure’: { # Configure argument
‘background’:’green’ # changing background argument
}
}
}
)
# For using the theme
style.theme_use(‘class_name’)
We created a style theme function with the help of ttk style. We use theme_create to create the functionality and can use that using theme_use for assigning the theme.
Below is the implementation:
Python3
import tkinter as tk
import tkinter.ttk as ttk
root = tk.Tk()
style = ttk.Style()
style = ttk.Style()
style.theme_create( 'style_class' ,
settings = {
'TLabelframe' : {
'configure' : {
'background' : 'green'
}
},
'TLabelframe.Label' : {
'configure' : {
'background' : 'green'
}
}
}
)
style.theme_use( 'style_class' )
labelframe = ttk.LabelFrame(root, text = "Group" )
labelframe.pack(padx = 30 , pady = 30 )
left = tk.Label(labelframe, text = "Geeks for Geeks" )
left.pack()
root.mainloop()
|
Output:
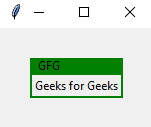
Please Login to comment...