How to Build a Simple Auto-Login Bot with Python
Last Updated :
19 Oct, 2021
In this article, we are going to see how to built a simple auto-login bot using python.
In this present scenario, every website uses authentication and we have to log in by entering proper credentials. But sometimes it becomes very hectic to login again and again to a particular website. So, to come out of this problem lets, built our own auto login bot using python.
We will be using Selenium (python library) for making the auto-login bot. Python Selenium library helps us to access all functionalities of Selenium WebDriver like Firefox, Chrome, Remote etc.
Installation
First of all, we have to install selenium using the below command:
pip install selenium
After successful installation of selenium, we also have to install chromedriver for accessing the chrome webdriver of selenium. You can download the same from here (Download version according to your system chrome version and according to your OS).
Make sure that you have noted the location where the chromedriver has been downloaded (as it is used in our python script). Now After downloading extract the zip file and please note the file location of the extracted file as we have needed it later in python code. (You can find the location by clicking on properties and then details).
Stepwise Implementation:
- First of all import the webdrivers from the selenium library.
- Find the URL of the login page to which you want to logged in.
- Provide the location executable chrome driver to selenium webdriver to access the chrome browser.
- Finally, find the name or id or class or CSS selector of username and password by right-clicking inspect on username and password.
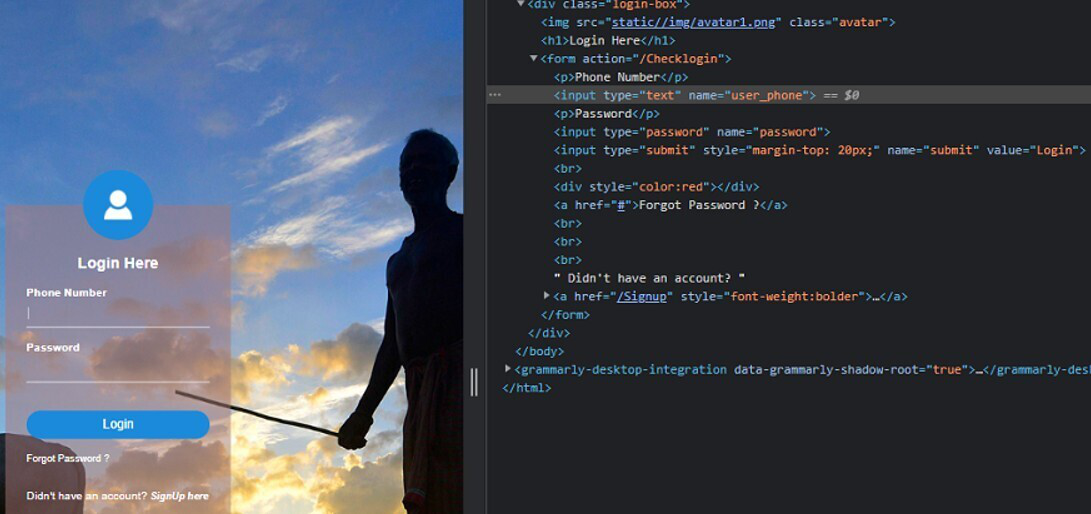
Below is the implementation:
Python3
from selenium import webdriver
import os
def startBot(username, password, url):
path = "C:\\Users\\hp\\Downloads\\chromedriver"
driver = webdriver.Chrome(path)
driver.get(url)
driver.find_element_by_name(
"id/class/name of username" ).send_keys(username)
driver.find_element_by_name(
"id/class/name of password" ).send_keys(password)
driver.find_element_by_css_selector(
"id/class/name/css selector of login button" ).click()
username = "Enter your username"
password = "Enter your password"
url = "Enter the URL of login page of website"
startBot(username, password, url)
|
Output:
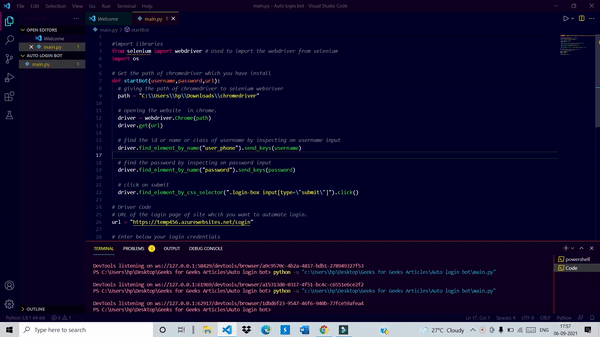
Please Login to comment...