Download Google Image Using Python and Selenium
Last Updated :
06 Mar, 2023
In this article, we are going to see how to download google Image using Python and Selenium.
Installation
On the terminal of your PC, type the following command. If it triggers any error regarding pip then you need to 1st install pip on windows manually by python get-pip.py command then you can run the following command.
pip install selenium
We also need to install a web driver that will help us to automatically run the web browser. You can install Firefox web driver, Internet Explorer web driver, or Chrome web driver. In this article, we will be using Chrome Web Driver.
The automation script interacts with the webpage by finding the element(s) we specified. There are various ways to find the elements in a webpage. The simplest way is to select the HTML tag of the desired element and copy its XPath. To do this, simply Right-Click on the webpage, click on “Inspect”, and copy the desired element’s XPath. You can also use the name or CSS of the element if you want to.
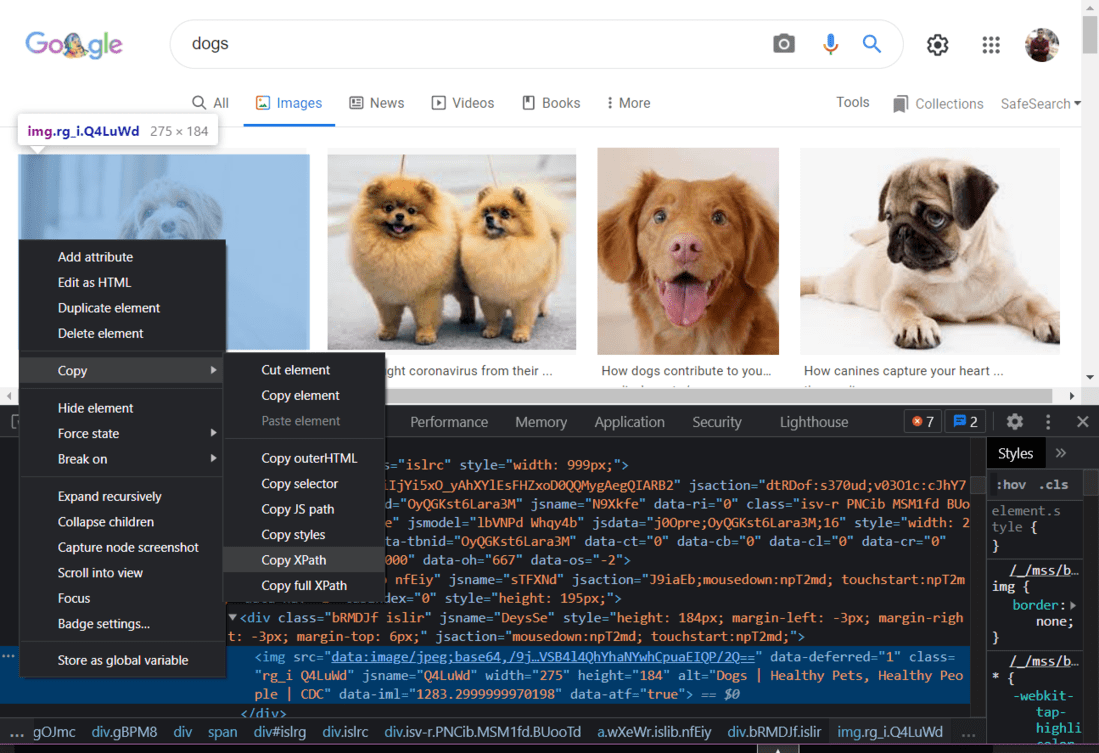
HTML of Google Images’ Result
Below is the implementation:
Python3
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
query = "dogs"
driver = webdriver.Chrome( 'Enter-Location-Of-Your-Webdriver' )
driver.maximize_window()
box = driver.find_element_by_xpath( '//*[@id="sbtc"]/div/div[2]/input' )
box.send_keys(query)
box.send_keys(Keys.ENTER)
def scroll_to_bottom():
last_height = driver.execute_script('\
return document.body.scrollHeight')
while True :
driver.execute_script('\
window.scrollTo( 0 ,document.body.scrollHeight)')
time.sleep( 3 )
new_height = driver.execute_script('\
return document.body.scrollHeight')
try :
driver.find_element_by_css_selector( ".YstHxe input" ).click()
time.sleep( 3 )
except :
pass
if new_height = = last_height:
break
last_height = new_height
scroll_to_bottom()
for i in range ( 1 , 50 ):
try :
img = driver.find_element_by_xpath(
'//*[@id="islrg"]/div[1]/div[' +
str (i) + ']/a[1]/div[1]/img' )
img.screenshot( 'Download-Location' +
query + ' (' + str (i) + ').png' )
time.sleep( 0.2 )
except :
continue
driver.close()
|
Result:
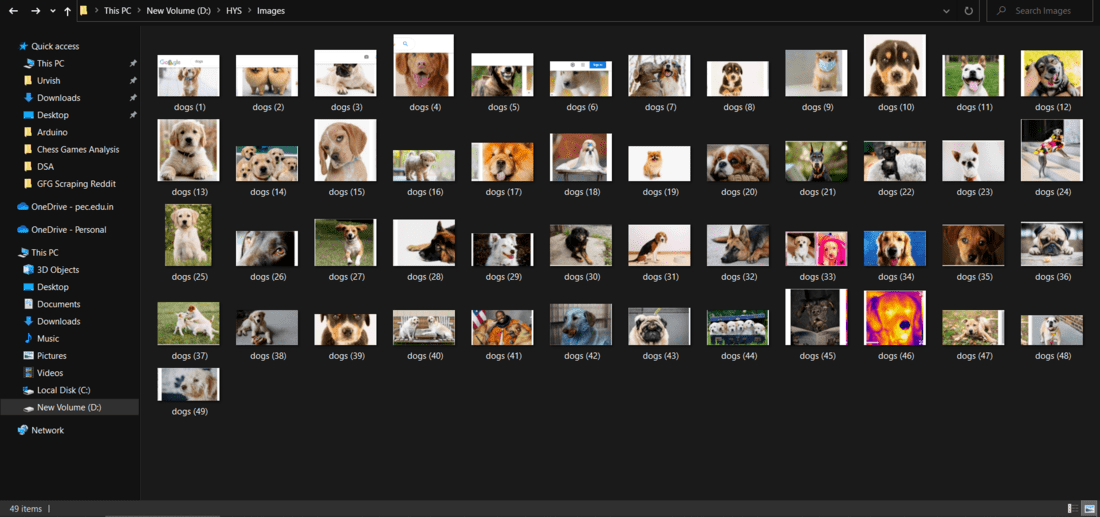
Captured Images
Well, this is the simplest way to create an automation script. This small program can be your fun little project. This could be the starting point of your journey with Selenium. You can use Selenium to do different things like scrape news from Google News. So keep your mind open about new ideas and you might end up creating a great project with Selenium and Python.
Please Login to comment...