How to switch to new window in Selenium for Python?
Last Updated :
15 Mar, 2021
Selenium is the most used Python tool for testing and automation for the web. But the problem occurs when it comes to a scenario where users need to switch between windows in chrome. Hence, selenium got that cover as well. Selenium uses these methods for this task-
- window_handles is used for working with different windows. It stores the window ids that are used for switching.
- switch_to.window method is used for switching between the windows with the help of window_handles ids.
Steps to cover:
- Set up the URL and import selenium
Python3
from selenium import webdriver
import time
PATH = "C:/chromedriver.exe"
driver = webdriver.Chrome(PATH)
|
- Get the website URL and click on the link which opens in a new window. And switch between them.
Python3
print ( "First window title = " + driver.title)
driver.find_element_by_class_name( "privacy" ).click()
time.sleep( 7 )
driver.switch_to.window(driver.window_handles[ 1 ])
print ( "Second window title = " + driver.title)
driver.switch_to.window(driver.window_handles[ 0 ])
print (driver.window_handles)
|
Output:
First window title = Yahoo
Second window title = Welcome to the Verizon Media Privacy Policy | Verizon Media Policies
['CDwindow-F25D48D2602CBD780FB2BE8B34A3BEAC', 'CDwindow-A80A74DFF7CCD47F628AF860F3D46913']
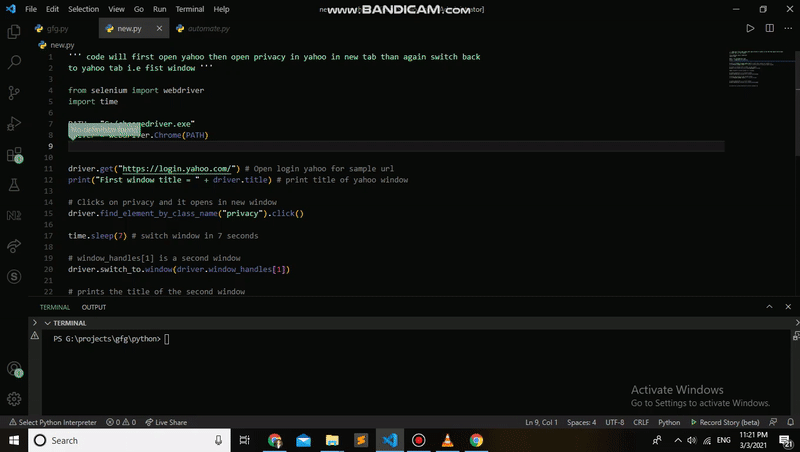
Explanation:
The program will first open yahoo then open privacy on yahoo in a new tab then again switch back to the yahoo tab in 7 seconds i.e first window.
Optional Case: If the user needs to open a new window and switch between them.
- execute_script is a method for passing JavaScript as a string
- window.open() is a method for opening the new window
Example 1:
Python3
driver.execute_script( "window.open()" )
driver.switch_to.window(driver.window_handles[ 2 ])
print (driver.title)
|
Output:
GeeksforGeeks | A computer science portal for geeks
Explanation:
The program enables to open a new window with get() method having parameter as geeks for geeks URL and prints the title of that window.
Example 2:
Python3
from selenium import webdriver
import time
PATH = "C:/chromedriver.exe"
driver = webdriver.Chrome(PATH)
print ( "First window title = " + driver.title)
driver.find_element_by_class_name( "privacy" ).click()
print (driver.window_handles)
driver.switch_to.window(driver.window_handles[ 1 ])
print ( "Second window title = " + driver.title)
driver.execute_script( "window.open()" )
print (driver.window_handles)
driver.switch_to.window(driver.window_handles[ 2 ])
print (driver.title)
|
Output:
First window title = Yahoo
[‘CDwindow-3D8EFAF1BC9A66342F78731C64C802BD’, ‘CDwindow-B6ADD61824FA954B7E52A9844D304C34’]
Second window title = Welcome to the Verizon Media Privacy Policy | Verizon Media Policies
[‘CDwindow-3D8EFAF1BC9A66342F78731C64C802BD’, ‘CDwindow-B6ADD61824FA954B7E52A9844D304C34’, ‘CDwindow-3176B13D77F832E4DEC6869134FECD1D’]
GeeksforGeeks | A computer science portal for geeks
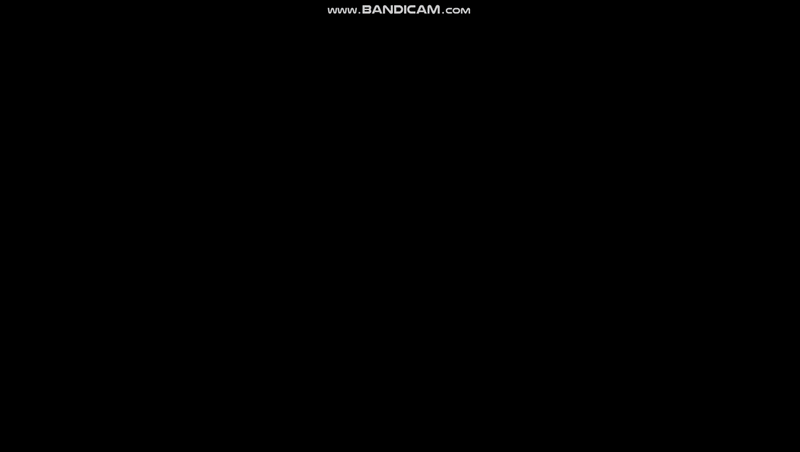
Explanation:
The script does the following:
- Opens yahoo and prints the title.
- Opens the privacy from yahoo and prints the title.
- Opens the new window and opens geeks for geeks on it and prints the title.
Please Login to comment...